计算周长是一个基本的数学问题,可以通过编程来实现。下面以Python语言为例,展示如何编写一个计算周长的程序。
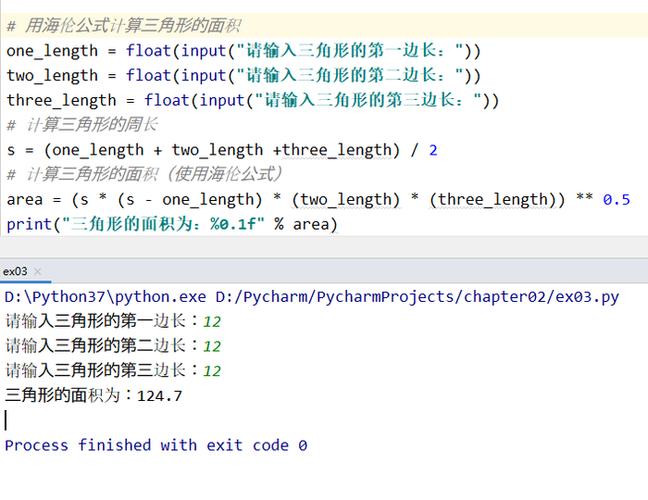
```python
def calculate_perimeter(shape, *args):
perimeter = 0
if shape == "rectangle" and len(args) == 2:
perimeter = 2 * (args[0] args[1])
elif shape == "square" and len(args) == 1:
perimeter = 4 * args[0]
elif shape == "circle" and len(args) == 1:
perimeter = 2 * 3.14 * args[0]
else:
print("Invalid input or shape!")
return perimeter
shape = input("Enter the shape (rectangle, square, circle): ")
if shape in ["rectangle", "square", "circle"]:
if shape == "rectangle":
length = float(input("Enter the length: "))
width = float(input("Enter the width: "))
result = calculate_perimeter(shape, length, width)
elif shape == "square":
side = float(input("Enter the side length: "))
result = calculate_perimeter(shape, side)
elif shape == "circle":
radius = float(input("Enter the radius: "))
result = calculate_perimeter(shape, radius)
print(f"The perimeter of the {shape} is: {result}")
else:
print("Invalid shape input!")
```
这段代码定义了一个函数`calculate_perimeter`来计算不同形状的周长,包括矩形、正方形和圆形。用户可以输入要计算的形状和相应的参数,程序将返回计算出的周长值。
通过这样的编程实现,可以方便快捷地计算各种形状的周长,提高工作效率。