Advanced Swift Programming
Swift is a powerful and versatile programming language developed by Apple for iOS, macOS, watchOS, and tvOS development. As you delve deeper into Swift programming, here are some advanced topics and techniques to enhance your skills:
Generics in Swift allow you to write flexible, reusable functions and data types that can work with any type. By using generics, you can write code that is more abstract and versatile, reducing code duplication and improving code maintainability.
Protocol-oriented programming is a paradigm in Swift that emphasizes protocol composition over class inheritance. By defining protocols and using protocol extensions, you can create composable and reusable code that promotes code reuse and modularity.
Swift provides several mechanisms for error handling, including throwing and catching errors, as well as the Result type. By mastering advanced error handling techniques, you can write more robust and reliable code that gracefully handles errors and failures.
Concurrency in Swift allows you to write code that performs multiple tasks simultaneously. By using techniques such as Grand Central Dispatch (GCD), Operation Queues, and async/await, you can write efficient and responsive code that takes full advantage of modern multi-core processors.
Swift uses Automatic Reference Counting (ARC) to manage memory automatically. However, understanding memory management principles such as strong, weak, and unowned references is crucial to prevent memory leaks and optimize performance in your Swift applications.
To create high-performance Swift applications, you need to optimize your code for speed and efficiency. Techniques such as lazy loading, value types, and minimizing memory allocations can help improve the performance of your Swift applications.
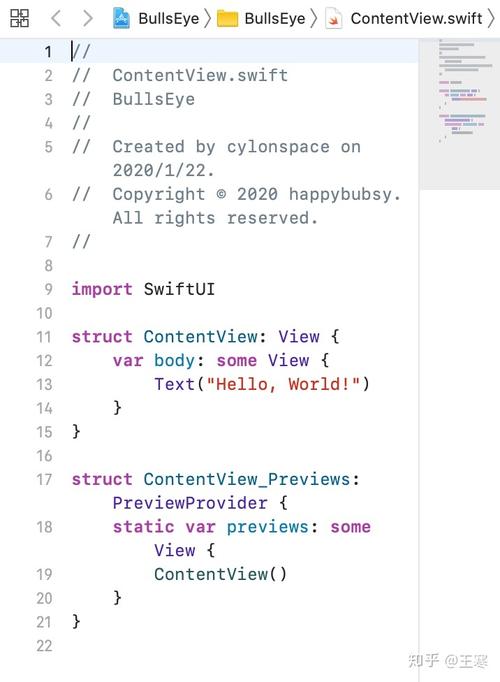
Explore advanced Swift libraries and frameworks that can streamline your development process and add powerful features to your applications. Libraries such as Alamofire for networking, Realm for data persistence, and RxSwift for reactive programming can take your Swift development to the next level.
Mastering debugging and profiling tools in Xcode can help you identify and fix issues in your Swift code more effectively. Learn how to use Xcode's debugging features, Instruments, and other profiling tools to optimize your code and improve the performance of your Swift applications.
By mastering these advanced Swift programming topics and techniques, you can become a more proficient and efficient Swift developer. Continuously learning and exploring new features of Swift will not only enhance your skills but also enable you to create innovative and high-quality applications for Apple platforms.