**Title: Understanding Regular Expressions in Programming**
Regular expressions, often abbreviated as regex or regexp, are powerful tools used in programming for pattern matching and text manipulation. Whether you're a beginner or an experienced programmer, understanding regular expressions can significantly enhance your ability to process and manipulate text efficiently. Let's delve into the world of regular expressions and explore their applications across various programming languages and industries.
### What are Regular Expressions?
At its core, a regular expression is a sequence of characters that define a search pattern. This pattern can be used to match strings or substrings within a larger text body. Regular expressions provide a concise and flexible means for identifying, capturing, and manipulating text data based on specific criteria.
### Basic Syntax
The syntax of regular expressions may vary slightly across different programming languages, but the fundamental concepts remain consistent. Here are some common elements:
1. **Literals**: Characters such as letters, digits, or symbols that match themselves.
- Example: The regular expression `hello` matches the string "hello" exactly.
2. **Metacharacters**: Special characters with predefined meanings in regular expressions.
- Examples:
- `.` (dot): Matches any single character except newline.
- `^`: Anchors the match to the start of the string.
- `$`: Anchors the match to the end of the string.
- `*`, ` `, `?`: Quantifiers specifying the number of occurrences.
- `\`: Escapes special characters to be treated as literals.
3. **Character Classes**: Define a set of characters to match a single character from that set.
- Example: `[aeiou]` matches any lowercase vowel.
4. **Grouping and Capturing**: Parentheses `()` are used to group patterns together and capture substrings for later use.
- Example: `(foo|bar)` matches either "foo" or "bar".
### Applications in Programming
Regular expressions find applications in various domains, including:
1. **Text Processing**: Searching, replacing, and extracting information from textual data.
2. **Form Validation**: Validating input fields such as email addresses, phone numbers, and zip codes.
3. **Web Scraping**: Extracting specific content from web pages by matching patterns.
4. **Data Extraction**: Parsing structured data formats like CSV, XML, and JSON.
5. **Log Analysis**: Filtering and analyzing log files to extract relevant information.
### Best Practices and Tips
To leverage regular expressions effectively, consider the following best practices:
1. **Keep it Simple**: Start with basic patterns and gradually add complexity as needed. Complex regular expressions can be difficult to understand and maintain.
2. **Testing and Validation**: Test your regular expressions against a variety of input data to ensure they behave as expected. Use online regex testers for quick validation.
3. **Documentation and Comments**: Document complex regular expressions with comments to explain their purpose and individual components.
4. **Performance Considerations**: Be mindful of performance implications, especially when dealing with large datasets. Inefficient regular expressions can impact the overall performance of your application.
5. **Learning Resources**: Invest time in learning regular expressions thoroughly. There are many online tutorials, books, and resources available to help you master this powerful tool.
### Conclusion
Regular expressions are indispensable tools for text processing and pattern matching in programming. By mastering regular expressions, you can significantly enhance your ability to manipulate textual data efficiently across various programming languages and applications. Start with the basics, practice regularly, and gradually explore more advanced features to become proficient in leveraging regular expressions effectively.
Remember, with great power comes great responsibility. Use regular expressions judiciously and always prioritize readability and maintainability in your code. Happy coding!
**References:**
- [Regular Expressions - MDN Web Docs](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Regular_Expressions)
- [Regular-Expressions.info - Regex Tutorial, Examples, and Reference](https://www.regular-expressions.info/)
- [Regex101 - Online Regular Expression Tester and Debugger](https://regex101.com/)
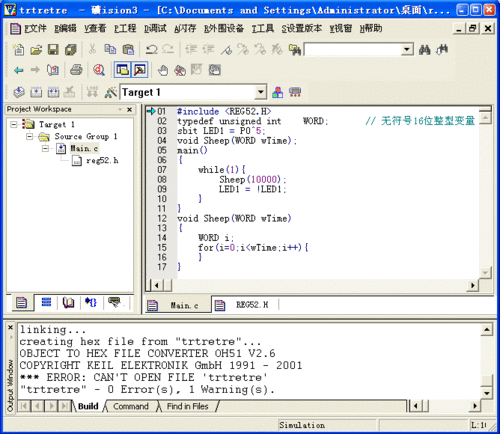
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。