Title: Exploring JDK Programming for Application Development
HTML:
```html
Exploring JDK Programming for Application Development
Java Development Kit (JDK) is a fundamental tool for Java developers, providing a collection of programming tools for developing Java applications. Let's delve into how JDK can be utilized for app development:
JDK encompasses the Java Runtime Environment (JRE), compilers, and various tools necessary for Java development. It enables developers to create Java applications, applets, and components. Understanding its components is crucial:
- JRE (Java Runtime Environment): Provides the libraries, Java Virtual Machine (JVM), and other components required to run Java applications.
- Compiler: Converts Java source code into bytecode, which can be executed by the JVM.
- Tools: JDK includes various tools such as javac (Java compiler), jar (Java archive tool), and javadoc (Java documentation generator).
Before diving into JDK programming, ensure you have JDK installed on your system. Follow these steps:
Once JDK is set up, you can start developing Java applications. Here's a basic example:
```java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
```
Save the above code in a file named HelloWorld.java
. Compile it using the command:
javac HelloWorld.java
This command will generate a bytecode file named HelloWorld.class
. Now, execute the program with:
java HelloWorld
You should see Hello, World!
printed in the console.
Beyond the basics, JDK offers a plethora of features to facilitate application development:
- Java APIs: Utilize the extensive Java API libraries for various functionalities like networking, database access, GUI development, etc.
- Integrated Development Environments (IDEs): IDEs like IntelliJ IDEA, Eclipse, and NetBeans provide advanced features for JDK programming, including code debugging, refactoring, and project management.
- Concurrency Utilities: JDK offers concurrency utilities like
java.util.concurrent
package for efficient multithreading and concurrent programming. - JavaFX: For desktop application development, JavaFX provides a rich set of UI controls and multimedia support.
- Security: JDK incorporates robust security features to develop secure applications, including encryption, authentication, and authorization mechanisms.
For efficient JDK programming and application development, consider the following best practices:
- Follow Java Naming Conventions: Adhere to Java naming conventions for classes, methods, variables, and packages to maintain code readability and consistency.
- Use Exception Handling: Implement proper exception handling to gracefully manage runtime errors and ensure application stability.
- Modularize Your Code: Break down your application into modular components for better maintainability, scalability, and reusability.
- Adopt Design Patterns: Familiarize yourself with common design patterns like Singleton, Factory, and MVC to solve recurring design problems effectively.
- Regularly Update JDK: Stay updated with the latest JDK releases to leverage new features, enhancements, and security patches.
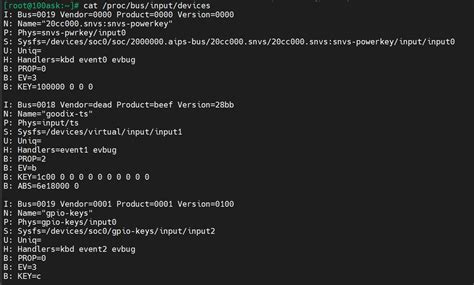
By mastering JDK programming techniques and incorporating best practices, you can develop robust, scalable, and secure Java applications tailored to your requirements.