Title: Getting Started with CFG Programming
Introduction to ContextFree Grammar (CFG) Programming
ContextFree Grammar (CFG) is a formalism used to describe the syntax of programming languages, natural languages, and various other formal languages. In the realm of computer science, CFG plays a crucial role in parsing and understanding the structure of programming languages. In this tutorial, we will provide a comprehensive introduction to CFG programming, covering its fundamentals, syntax, applications, and practical examples.
1. Understanding ContextFree Grammar
ContextFree Grammar consists of a set of production rules that describe the syntax of a language. It comprises four components:
Terminals: The basic symbols of the language.
Nonterminals: Symbols representing groups of terminals.
Production rules: Define how nonterminals can be replaced by combinations of terminals and other nonterminals.
Start symbol: The initial nonterminal from which the derivation of strings begins.
2. Basic Syntax of CFG
A CFG is typically denoted as G = (V, Σ, R, S), where:
V is a set of nonterminal symbols.
Σ is a set of terminal symbols.
R is a set of production rules.
S is the start symbol.
3. Example CFG
Let's consider a simple CFG for arithmetic expressions:
V = {Expr, Term, Factor}
Σ = {a, , *, (, )}
R:
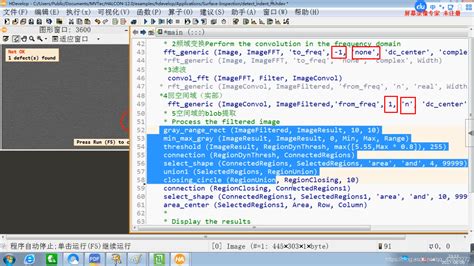
Expr > Expr Term | Term
Term > Term * Factor | Factor
Factor > ( Expr ) | a
S = Expr
4. CFG Parsing
Parsing is the process of analyzing a string of symbols to determine its structure with respect to a given CFG. There are different parsing techniques, such as:
Topdown parsing (e.g., Recursive Descent Parsing)
Bottomup parsing (e.g., ShiftReduce Parsing)
5. Applications of CFG
CFG has various applications in computer science:
Compiler design: CFG is used in lexical analysis and parsing phases of compilers to recognize the syntax of programming languages.
Natural language processing: CFG is employed to model the syntax of natural languages for tasks like parsing and language generation.
Data validation: CFG can be used to define the structure of data formats such as XML and JSON for validation purposes.
6. Practical Example: Parsing Arithmetic Expressions
Let's write a simple Python program to parse arithmetic expressions using a CFG:
```python
Define the CFG rules
rules = {
"Expr": ["Expr Term", "Term"],
"Term": ["Term * Factor", "Factor"],
"Factor": ["( Expr )", "a"]
}
Function to parse expression
def parse(input_str, symbol):
if input_str == "":
return True
if symbol not in rules:
return False
for rule in rules[symbol]:
tokens = rule.split()
if all(parse(input_str[i:], tokens[i]) for i in range(len(tokens))):
return True
return False
Main function
def main():
expression = input("Enter an arithmetic expression: ")
if parse(expression, "Expr"):
print("Valid expression!")
else:
print("Invalid expression!")
if __name__ == "__main__":
main()
```
Conclusion
ContextFree Grammar (CFG) is a fundamental concept in computer science, widely used in various applications such as compiler design, natural language processing, and data validation. By understanding CFG and its principles, programmers can effectively parse and analyze the syntax of languages, leading to the development of robust software systems.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。