Title: Exploring AVX Programming: A Practical Example
Introduction to AVX:
AVX (Advanced Vector Extensions) is a set of CPU instructions introduced by Intel to improve the performance of certain types of computations, particularly those involving SIMD (Single Instruction, Multiple Data) operations. AVX allows for parallel processing of multiple data elements simultaneously, which can significantly accelerate certain computational tasks, such as multimedia processing, scientific simulations, and data analysis.
Understanding SIMD:
Before diving into AVX programming, it's essential to understand the concept of SIMD. SIMD allows a single instruction to operate on multiple data elements in parallel. This is particularly useful in scenarios where the same operation needs to be performed on a large set of data, such as adding two arrays together or multiplying matrices.
Practical Example: Vector Addition using AVX:
Let's consider a simple example of adding two vectors using AVX instructions. Suppose we have two arrays of floatingpoint numbers, `float array1[N]` and `float array2[N]`, and we want to compute the elementwise sum and store the result in a third array, `float result[N]`.
Step 1: Initialization:
First, we need to initialize the arrays with some sample data. This step is crucial for testing our AVX implementation.
```c
include
define N 1024 // Array size
void vector_add(float* array1, float* array2, float* result, int n) {
// Loop over array elements
for (int i = 0; i < n; i) {
result[i] = array1[i] array2[i]; // Perform elementwise addition
}
}
int main() {
float array1[N], array2[N], result[N];
// Initialize arrays with sample data
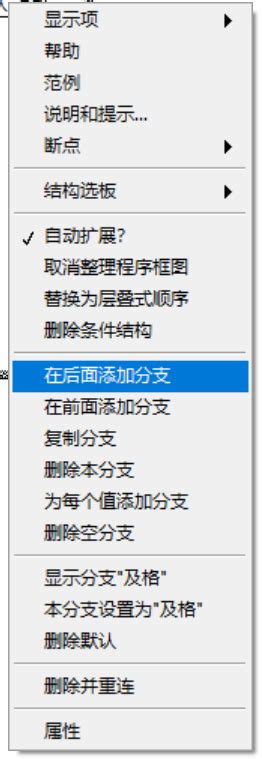
for (int i = 0; i < N; i) {
array1[i] = i;
array2[i] = 2 * i;
}
// Perform vector addition
vector_add(array1, array2, result, N);
// Output the result (for verification)
for (int i = 0; i < N; i) {
printf("%f ", result[i]);
}
return 0;
}
```
Step 2: Vectorization using AVX:
Now, let's optimize the `vector_add` function using AVX instructions to leverage SIMD parallelism.
```c
void vector_add_avx(float* array1, float* array2, float* result, int n) {
// Loop over array elements in steps of 8 (AVX can process 8 floats at once)
for (int i = 0; i < n; i = 8) {
// Load 8 floats from array1 and array2 into AVX registers
__m256 vec1 = _mm256_loadu_ps(&array1[i]);
__m256 vec2 = _mm256_loadu_ps(&array2[i]);
// Add the vectors elementwise
__m256 sum = _mm256_add_ps(vec1, vec2);
// Store the result back to memory
_mm256_storeu_ps(&result[i], sum);
}
}
```
Step 3: Testing and Verification:
Finally, we need to test our optimized `vector_add_avx` function to ensure correctness and measure performance improvements.
```c
int main() {
float array1[N], array2[N], result[N];
// Initialize arrays with sample data
for (int i = 0; i < N; i) {
array1[i] = i;
array2[i] = 2 * i;
}
// Perform vector addition using AVX
vector_add_avx(array1, array2, result, N);
// Output the result (for verification)
for (int i = 0; i < N; i) {
printf("%f ", result[i]);
}
return 0;
}
```
Conclusion:
In this example, we explored how to utilize AVX instructions for vector addition, a common SIMD operation. By leveraging AVX, we were able to significantly improve the performance of our code by parallelizing the addition operation. AVX programming opens up opportunities for optimizing performancecritical applications across various domains, from scientific computing to multimedia processing.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。