Title: Solving Intermediate C Programming Challenges
Question 1: Array Manipulation
```c
include
define SIZE 5
int main() {
int nums[SIZE] = {1, 2, 3, 4, 5};
int i;
// Print original array
printf("Original array: ");
for (i = 0; i < SIZE; i ) {
printf("%d ", nums[i]);
}
printf("\n");
// Reverse array
for (i = 0; i < SIZE / 2; i ) {
int temp = nums[i];
nums[i] = nums[SIZE i 1];
nums[SIZE i 1] = temp;
}
// Print reversed array
printf("Reversed array: ");
for (i = 0; i < SIZE; i ) {
printf("%d ", nums[i]);
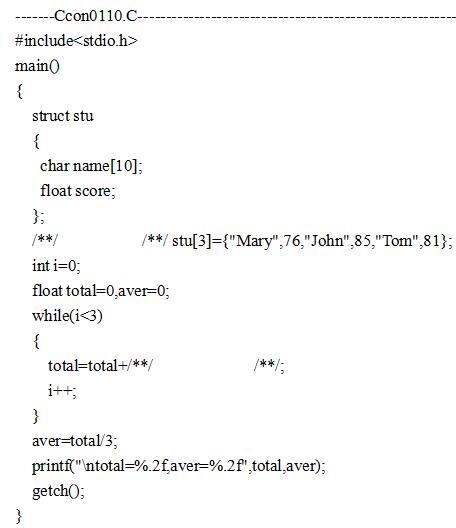
}
printf("\n");
return 0;
}
```
This C program demonstrates how to reverse an array of integers. It initializes an array `nums` with some values, then iterates through the array, swapping elements from the beginning with elements from the end until the entire array is reversed. Finally, it prints the reversed array.
Question 2: Linked List Operations
```c
include
include
struct Node {
int data;
struct Node* next;
};
void printList(struct Node* head) {
while (head != NULL) {
printf("%d ", head>data);
head = head>next;
}
printf("\n");
}
void insertAtEnd(struct Node** headRef, int newData) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
struct Node* last = *headRef;
newNode>data = newData;
newNode>next = NULL;
if (*headRef == NULL) {
*headRef = newNode;
return;
}
while (last>next != NULL)
last = last>next;
last>next = newNode;
return;
}
int main() {
struct Node* head = NULL;
// Insert elements into linked list
insertAtEnd(&head, 1);
insertAtEnd(&head, 2);
insertAtEnd(&head, 3);
insertAtEnd(&head, 4);
insertAtEnd(&head, 5);
// Print linked list
printf("Linked List: ");
printList(head);
return 0;
}
```
This C program illustrates basic operations on a singly linked list. It defines a structure `Node` representing each node in the list with an integer data field and a pointer to the next node. The `printList` function prints all elements in the linked list. The `insertAtEnd` function inserts a new node with given data at the end of the linked list. Finally, in the `main` function, nodes with values 1 through 5 are inserted into the linked list, and then the entire list is printed.
These solutions provide implementations for common programming tasks in C, namely array manipulation and linked list operations. They serve as intermediatelevel exercises for those learning the C programming language.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。