Title: Understanding and Implementing the flipud Function in Programming
In programming, the `flipud` function is a common operation used in various contexts, particularly in array manipulation or image processing. This function flips the elements of an array or a matrix vertically, essentially reversing their order along the rows. Let's delve into understanding its significance, implementation, and potential applications.
Understanding the flipud Function:
The `flipud` function is primarily used to flip the elements of an array or a matrix vertically. It flips the order of rows along the vertical axis, effectively reversing the sequence of elements from top to bottom.
Implementation in Different Programming Languages:
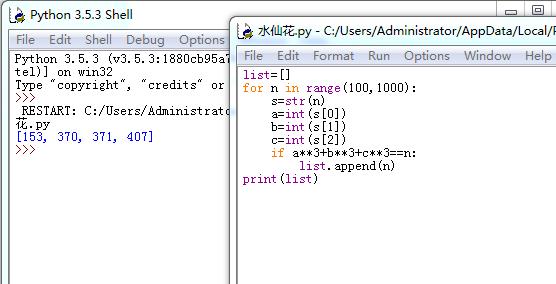
Python:
```python
import numpy as np
Define an array
arr = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
Using flipud function
flipped_arr = np.flipud(arr)
print("Original array:")
print(arr)
print("\nArray after flipping vertically:")
print(flipped_arr)
```
MATLAB:
```matlab
% Define a matrix
mat = [1 2 3; 4 5 6; 7 8 9];
% Using flipud function
flipped_mat = flipud(mat);
disp('Original matrix:')
disp(mat)
disp('Matrix after flipping vertically:')
disp(flipped_mat)
```
JavaScript (using TensorFlow.js):
```javascript
const tf = require('@tensorflow/tfjsnode');
// Define a tensor
const tensor = tf.tensor([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]]);
// Using flipud function
const flippedTensor = tensor.reverse(0);
console.log('Original tensor:');
tensor.print();
console.log('Tensor after flipping vertically:');
flippedTensor.print();
```
Applications:
1.
Image Processing:
In image processing tasks, flipping images vertically might be required for various transformations or data augmentation techniques.2.
Data Analysis:
When dealing with datasets represented as matrices or arrays, flipping data vertically can sometimes provide insights or meet specific analytical requirements.3.
Game Development:
In game development, flipping sprites or game elements vertically is a common operation, especially for character animations or sprite sheets.4.
Signal Processing:
In signal processing applications, flipping signals or waveforms vertically might be necessary for certain operations or analysis.Guidance for Usage:
Efficiency Consideration:
While the `flipud` function is convenient, depending on the size of the array or matrix, its usage might impact performance. Consider alternatives or optimizations if dealing with large datasets.
Integration with Libraries:
Many programming languages offer builtin functions for array manipulation. Explore the available libraries or modules to leverage optimized implementations of `flipud` and related functions.
Documentation:
Always refer to the documentation of the programming language or library you are using for specific details on the `flipud` function's behavior and parameters.In conclusion, the `flipud` function plays a crucial role in array manipulation and image processing tasks, offering a simple yet powerful way to reverse the order of elements along the vertical axis. Understanding its implementation and applications can significantly enhance programming capabilities across various domains.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。