编程中求字符长度的函数
```html
body {
fontfamily: Arial, sansserif;
lineheight: 1.6;
padding: 20px;
}
h1 {
color: 333;
}
p {
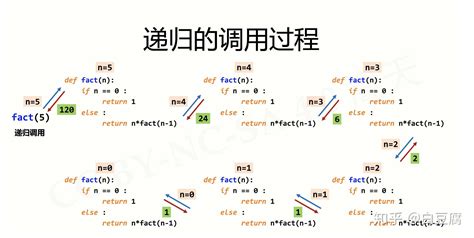
color: 666;
}
Understanding Incremental Functions in Programming
In programming, an incremental function refers to a function that increases its output value based on the input provided. These functions play a crucial role in various algorithms, applications, and software development processes. Let's delve deeper into the concept of incremental functions and their significance:
An incremental function, often referred to as an incremental algorithm or process, is a function that increases its output value by a fixed amount or in a systematic manner with each iteration or input provided. These functions are commonly used in scenarios where successive steps need to be taken to achieve a particular outcome or where values need to be incremented based on specific conditions.
There are numerous examples of incremental functions across different programming paradigms:
counter = 0
counter = 1 Increment the counter
print(counter) Output: 1
counter = 1 Increment the counter again
print(counter) Output: 2
- Progress Bar: In graphical user interfaces (GUIs), progress bars often represent incremental functions where the progress value increases gradually until a task is completed.
- Loop Iterations: Iterative constructs like loops frequently use incremental functions to control the flow of execution. For example, in a for loop, the loop variable is often incremented or decremented:
for i in range(5):
print(i) Output: 0, 1, 2, 3, 4
Incremental functions are essential in programming for several reasons:
- Efficiency: In many algorithms, incrementing values or progressing step by step is more efficient and practical than making significant jumps or changes.
- Controlled Progression: Incremental functions provide a structured way to control the progression of tasks or operations, ensuring that each step is completed before moving to the next.
- Scalability: By incrementing values systematically, incremental functions facilitate scalability and adaptability, allowing software to handle varying input sizes or conditions.
- Clarity: Using incremental functions often leads to clearer and more understandable code, as the logic of progression is explicit and easy to follow.
When working with incremental functions in programming, consider the following best practices:
- Choose Appropriate Data Types: Select data types that can accurately represent incremental values without loss of precision or overflow issues.
- Ensure Termination: In iterative algorithms, ensure that incremental functions lead to termination conditions to prevent infinite loops.
- Handle Boundary Cases: Pay attention to boundary cases where increments may lead to unexpected behavior or errors, and handle them appropriately.
- Optimize Performance: Optimize the performance of incremental functions by minimizing unnecessary operations and leveraging builtin language features or libraries.
Incremental functions are fundamental elements in programming, providing a structured approach to incrementing values and controlling progression in algorithms and applications. By understanding the concept of incremental functions and following best practices, developers can create efficient, scalable, and maintainable software solutions.