Understanding the Logic Behind Programming
Programming logic is the foundation of software development, dictating how a program operates and processes data. It's essentially the set of rules and principles that govern the flow of execution in a computer program. Let's delve into the core concepts of programming logic:
1. Sequential Execution:
Programs are executed line by line, in the order they are written.
Sequential execution implies that one statement is executed after the other, without any branching or looping.
2. Conditional Statements:
Conditional statements allow programs to make decisions based on certain conditions.
Common constructs include `if`, `else if`, and `else`.
Example:
```python
if condition:
code to execute if condition is True
else:
code to execute if condition is False
```
3. Looping Constructs:
Loops allow for repetitive execution of a block of code.
Common loop types include `for` loops and `while` loops.
Example (using a `for` loop in Python):
```python
for i in range(5):
code to repeat 5 times
```
4. Functions and Methods:
Functions encapsulate a block of code that can be reused throughout a program.
Methods are functions associated with objects in objectoriented programming.
Example (defining a function in Python):
```python
def greet(name):
print("Hello, " name)
calling the function
greet("John")
```
5. Recursion:
Recursion is a technique where a function calls itself in order to solve smaller instances of the same problem.
It's useful for tasks that can be broken down into smaller, similar subproblems.
Example (calculating factorial recursively in Python):
```python
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n1)
result = factorial(5)
```
6. Error Handling:
Error handling mechanisms, like `try` and `except` blocks, allow programs to gracefully handle unexpected situations.
They prevent crashes and help in debugging.
Example (handling division by zero in Python):
```python
try:
result = x / y
except ZeroDivisionError:
print("Error: Division by zero!")
```
7. Data Structures and Algorithms:
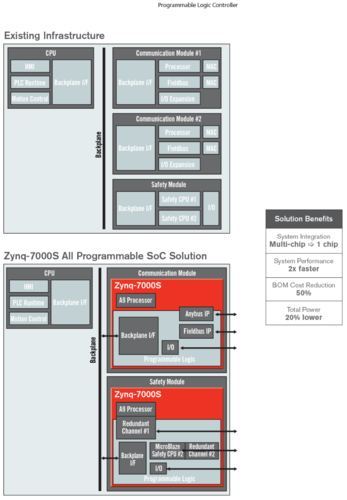
Understanding data structures (e.g., arrays, lists, stacks, queues) and algorithms (e.g., sorting, searching) is crucial for efficient programming.
They determine how data is stored, retrieved, and manipulated.
Example (implementing bubble sort in Python):
```python
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, ni1):
if arr[j] > arr[j 1]:
arr[j], arr[j 1] = arr[j 1], arr[j]
```
Conclusion:
Programming logic forms the backbone of any software system. Mastering these fundamental concepts empowers developers to write efficient, scalable, and maintainable code. Continuously honing your understanding of programming logic will enable you to tackle increasingly complex problems and contribute effectively to the world of software development.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。