Title: Mastering Precision Programming in MATLAB
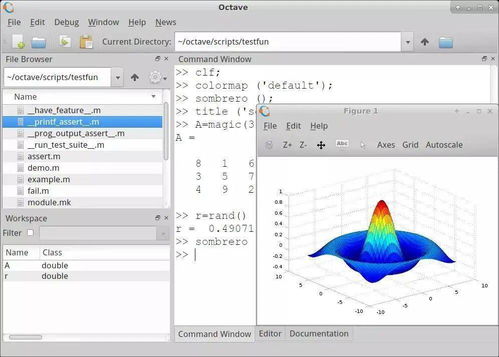
MATLAB, renowned for its versatility in numerical computing and data analysis, excels in handling complex mathematical operations. However, ensuring precision in MATLAB programming is crucial, especially in scientific and engineering applications where accuracy is paramount. This guide delves into the intricacies of precision programming in MATLAB, offering insights and best practices to enhance accuracy and reliability.
Precision in MATLAB refers to the degree of accuracy in numerical computations. MATLAB uses finite precision arithmetic, meaning that it represents real numbers with finite binary approximations. While this facilitates efficient computation, it also introduces limitations, such as roundoff errors and numerical instability.
1. Choose Appropriate Data Types:
Selecting the right data types is fundamental for precision programming in MATLAB. Use `double` for doubleprecision floatingpoint arithmetic, offering higher precision compared to `single`. For integer operations, opt for `int64` or `uint64` for enhanced accuracy.
```matlab
% Example: Define variables with specific data types
x = double(0.1);
y = int64(1234567890123456789);
```
2. Control Roundoff Errors:
Roundoff errors occur due to the finite precision representation of numbers. Minimize these errors by avoiding subtractive cancellation, especially in iterative computations. Utilize functions like `round`, `ceil`, or `floor` judiciously to control rounding behavior.
```matlab
% Example: Controlling roundoff errors
result = round(sqrt(2)^2 2, 10); % Round to 10 decimal places
```
3. Handle Numerical Instabilities:
Numerical instability arises when algorithms amplify errors during computation, leading to inaccurate results. Employ stable algorithms and numerical techniques such as preconditioning, regularization, and iterative refinement to mitigate instability issues.
```matlab
% Example: Using iterative refinement
x = A\b; % Direct solution
x_refined = lsqminnorm(A, b); % Iterative refinement
```
4. Utilize Symbolic Math Toolbox:
For symbolic computations requiring high precision, leverage MATLAB's Symbolic Math Toolbox. Symbolic variables and expressions maintain exact precision, eliminating numerical approximations inherent in floatingpoint arithmetic.
```matlab
% Example: Symbolic computation
syms x;
f = sin(x)^2 cos(x)^2; % Exact representation
```
While enhancing precision is crucial, it's essential to balance it with computational efficiency, especially for largescale simulations or realtime applications. Consider the following strategies to optimize performance:
Vectorization:
Utilize MATLAB's vectorized operations to exploit hardware parallelism and accelerate computations.
Preallocation:
Preallocate arrays to avoid dynamic memory reallocation, optimizing memory usage and computational speed.
Algorithmic Efficiency:
Optimize algorithms for computational complexity to reduce execution time without sacrificing precision.Precision programming in MATLAB demands a meticulous approach to ensure accurate and reliable results. By adhering to best practices, controlling errors, and leveraging appropriate tools, MATLAB users can achieve superior precision in numerical computations. Moreover, optimizing performance while maintaining precision facilitates efficient execution of complex algorithms across diverse scientific and engineering domains.
Mastering precision programming empowers MATLAB practitioners to tackle challenging problems with confidence, advancing research, innovation, and problemsolving capabilities.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。