Title: Understanding and Implementing While Loops in Programming
Introduction to While Loops:
While loops are fundamental constructs in programming, allowing the execution of a block of code repeatedly as long as a specified condition is true. They offer flexibility and efficiency in automating tasks and iterating over data. Let's delve into the details of while loops and how they are utilized across various programming languages.
Basic Syntax:
The syntax of a while loop typically consists of:
```
while (condition) {
// code block to be executed
}
```
Here, "condition" is evaluated before each iteration. If the condition is true, the code block inside the loop is executed. This process continues until the condition becomes false.
Key Components of While Loops:
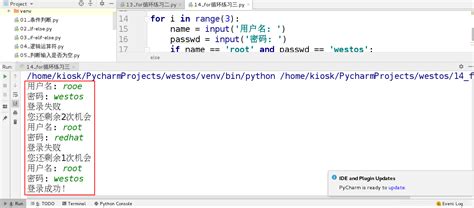
1.
Initialization:
Before entering a while loop, variables involved in the loop condition must be initialized appropriately.2.
Condition:
The condition is evaluated before each iteration. It determines whether the loop should continue executing or terminate.3.
Iteration:
After executing the code block inside the loop, the program revisits the condition. If it's still true, the loop repeats; otherwise, the loop terminates.Example in Python:
```python
count = 0
while count < 5:
print("Count is:", count)
count = 1
```
This Python code will print numbers from 0 to 4, as long as the count is less than 5.
Example in C:
```c
include
int main() {
int count = 0;
while (count < 5) {
printf("Count is: %d\n", count);
count ;
}
return 0;
}
```
Similarly, this C code achieves the same result.
Best Practices and Tips:
1.
Ensure Loop Termination:
Always ensure that the loop's condition will eventually become false to avoid infinite loops, which can crash programs.2.
Initialize Variables Properly:
Initialize loop control variables outside the loop and update them inside to maintain clarity and avoid unexpected behavior.3.
Use Caution with Infinite Loops:
Be cautious when implementing while loops, especially when the termination condition depends on user input or external factors.4.
Consider Alternative Constructs:
In some cases, for loops or higherlevel constructs might offer clearer logic and readability compared to while loops.Conclusion:
While loops are indispensable tools in programming, offering a powerful mechanism for iteration and automation. Understanding their syntax, usage, and best practices is essential for writing efficient and bugfree code. Whether you're a beginner or an experienced programmer, mastering while loops will significantly enhance your ability to tackle a wide range of programming tasks.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。