Title: A Beginner's Guide to Programming Summation
Programming is a powerful tool that enables us to automate tasks and solve complex problems efficiently. One common task in programming is calculating the sum of a series of numbers. Whether you're a beginner or an experienced programmer, understanding different methods for summing numbers is essential. In this guide, we'll explore various programming languages and techniques for summing numbers, providing you with a solid foundation to tackle summation in your coding projects.
1. Using Python:
Python is a versatile and beginnerfriendly programming language known for its simplicity and readability. Calculating the sum of numbers in Python can be done using builtin functions or loops.
```python
Using builtin sum() function
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print("Sum using builtin sum() function:", total)
Using a loop
total = 0
for num in numbers:
total = num
print("Sum using a loop:", total)
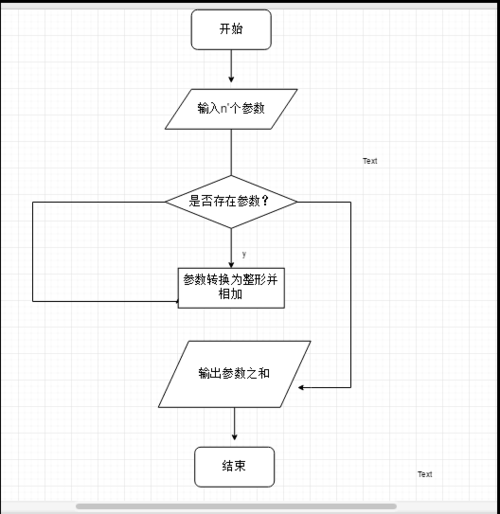
```
2. Using Java:
Java is a widelyused programming language known for its portability and versatility. In Java, you can sum numbers using loops or streams.
```java
// Using a loop
int[] numbers = {1, 2, 3, 4, 5};
int total = 0;
for (int num : numbers) {
total = num;
}
System.out.println("Sum using a loop: " total);
// Using streams (Java 8 )
int sum = Arrays.stream(numbers).sum();
System.out.println("Sum using streams: " sum);
```
3. Using JavaScript:
JavaScript is a popular programming language primarily used for web development. You can sum numbers in JavaScript using loops or array.reduce() method.
```javascript
// Using a loop
const numbers = [1, 2, 3, 4, 5];
let total = 0;
for (let i = 0; i < numbers.length; i ) {
total = numbers[i];
}
console.log("Sum using a loop:", total);
// Using array.reduce() method
const sum = numbers.reduce((acc, curr) => acc curr, 0);
console.log("Sum using array.reduce():", sum);
```
4. Using C:
C is a powerful programming language often used for system programming and embedded development. You can sum numbers in C using loops.
```c
include
int main() {
int numbers[] = {1, 2, 3, 4, 5};
int total = 0;
for (int i = 0; i < sizeof(numbers) / sizeof(numbers[0]); i ) {
total = numbers[i];
}
printf("Sum using a loop: %d\n", total);
return 0;
}
```
5. Tips for Efficient Summation:
Use Builtin Functions:
Whenever possible, leverage builtin functions provided by the programming language to perform summation. These functions are often optimized for efficiency.
Optimize Loops:
If you're summing a large number of elements, consider optimizing your loops to reduce computational overhead. For example, in languages like C or C , preallocating memory and minimizing unnecessary operations can improve performance.
Consider Parallelization:
For extremely large datasets, explore techniques such as parallel computing to distribute the summation across multiple processors or cores, thereby reducing processing time.Conclusion:
Summation is a fundamental operation in programming, and understanding how to efficiently calculate the sum of numbers is crucial for writing efficient code. By familiarizing yourself with various programming languages and techniques for summation, you'll be better equipped to tackle a wide range of coding challenges. Whether you're working with Python, Java, JavaScript, C, or any other programming language, the principles of summation remain consistent, empowering you to write cleaner, more efficient code.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。