Title: Exploring Ellipses in Programming
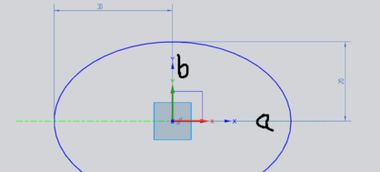
```html
body {
fontfamily: Arial, sansserif;
lineheight: 1.6;
margin: 0 auto;
maxwidth: 800px;
padding: 20px;
}
h1 {
color: 333;
}
p {
marginbottom: 20px;
}
Exploring Ellipses in Programming
Ellipses are fundamental geometric shapes in mathematics and computer graphics. In programming, understanding how to work with ellipses can be essential for tasks ranging from drawing shapes on a screen to modeling orbits in space. Let's delve into the world of ellipses in programming.
An ellipse is a closed curve in a plane that is symmetric about two perpendicular axes. Mathematically, an ellipse is defined as the set of all points for which the sum of the distances to two fixed points (the foci) is constant. The longest diameter of an ellipse is called the major axis, and the shortest diameter is called the minor axis.
In programming, ellipses can be represented in various ways, depending on the context:
- Mathematical Representation: In mathematical terms, an ellipse can be represented by its equation in the Cartesian coordinate system. The general form of the equation for an ellipse centered at the origin is: (x^2 / a^2) (y^2 / b^2) = 1, where a and b are the lengths of the semimajor and semiminor axes, respectively.
- Graphics Libraries: Most programming languages offer graphics libraries that provide functions or methods for drawing ellipses. These libraries often allow developers to specify the center, radii, and other parameters of the ellipse.
- Parametric Representation: Ellipses can also be represented parametrically, where the position of each point on the ellipse is described by a pair of parametric equations in terms of a parameter, such as angle or time.
Here's a basic example of how you might draw an ellipse using a popular graphics library like Canvas in HTML5:
<canvas id="myCanvas" width="400" height="200"></canvas><script>
var canvas = document.getElementById('myCanvas');
var context = canvas.getContext('2d');
var centerX = canvas.width / 2;
var centerY = canvas.height / 2;
var radiusX = 100;
var radiusY = 50;
context.beginPath();
context.ellipse(centerX, centerY, radiusX, radiusY, 0, 0, 2 * Math.PI);
context.stroke();
</script>
This code will draw an ellipse on an HTML canvas element with a width of 400 pixels and a height of 200 pixels. The ellipse will be centered on the canvas, with a horizontal radius of 100 pixels and a vertical radius of 50 pixels.
The versatility of ellipses makes them useful in various programming applications, including:
- Computer Graphics: Ellipses are commonly used to draw shapes, such as circles, ovals, and arcs, in graphical user interfaces and games.
- Physics Simulations: Ellipses are used to model the orbits of celestial bodies in physics simulations, such as planetary motion.
- Data Visualization: Ellipses can represent data distributions and confidence intervals in statistical charts and graphs.
Understanding ellipses and how to work with them in programming opens up a wide range of possibilities for creating visualizations, simulations, and applications in various domains. Whether you're drawing shapes on a screen or modeling celestial mechanics, the humble ellipse remains a powerful tool in the programmer's arsenal.