Understanding the Read Function in Programming
In programming, the `read()` function is a commonly used method for reading data from a particular source, such as a file or a stream. This function differs slightly in its implementation across various programming languages, but its fundamental purpose remains consistent: to retrieve data from a specified location. Let's delve into the usage and functionality of the `read()` function across different programming languages and contexts.
1. Python:
In Python, the `read()` function is primarily used in file handling operations. When applied to a file object, it reads a specified number of bytes from the file. If no argument is provided, it reads the entire file. Here's a basic usage example:
```python
Opening a file in read mode
file = open("example.txt", "r")
Reading the entire file
data = file.read()
print(data)
Closing the file
file.close()
```
In this example, `file.read()` reads the entire contents of the file "example.txt" and stores it in the variable `data`.
2. JavaScript:
JavaScript doesn't have a builtin `read()` function for file handling like Python. Instead, it utilizes different methods for reading data, such as `fetch()` for reading from URLs or `FileReader` for reading files in the browser environment. Here's a simplified example using `fetch()`:
```javascript
// Fetching data from a URL
fetch('https://api.example.com/data')
.then(response => response.text())
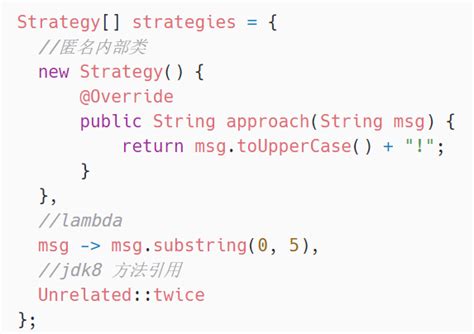
.then(data => {
console.log(data); // Output the fetched data
})
.catch(error => console.error('Error:', error));
```
In this JavaScript example, `fetch()` is used to retrieve data from a specified URL. The `then()` method is then used to handle the asynchronous response and extract the text data from it.
3. C :
In C , the `read()` function is part of the input/output stream library (`iostream`). It reads a specified number of characters from an input stream and stores them into an array or buffer. Here's a simple example:
```cpp
include
include
int main() {
std::ifstream file("example.txt"); // Open file in read mode
if (file.is_open()) {
char buffer[255]; // Buffer to store data
file.read(buffer, 255); // Read up to 255 characters
std::cout << buffer << std::endl; // Output the read data
file.close(); // Close the file
} else {
std::cerr << "Unable to open file" << std::endl;
}
return 0;
}
```
In this C example, `file.read(buffer, 255)` reads up to 255 characters from the file "example.txt" and stores them into the buffer. Finally, the data is output using `std::cout`.
Guidance and Best Practices:
Ensure proper error handling when using the `read()` function to handle unexpected situations such as file not found or network errors.
Always close file streams after reading to free up system resources and prevent potential memory leaks.
Be mindful of the amount of data being read, especially when dealing with large files, to avoid performance issues and memory consumption.
Understanding the `read()` function and its usage in different programming languages equips developers with the necessary tools to efficiently read and process data from various sources. Whether it's reading from files, URLs, or input streams, mastering this function is essential for effective data manipulation and application development.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。