编程最难的是什么语言
Title: Practical Python Programming Solutions
Practical Python Programming Solutions
Python is a versatile programming language used across various industries and domains. Whether you're a beginner or an experienced developer, here are some practical solutions to common programming challenges in Python:
When working with data, Python offers powerful libraries like Pandas and NumPy. For instance, to read a CSV file into a DataFrame and perform basic analysis:
```python
import pandas as pd
Read CSV file into DataFrame
data = pd.read_csv('data.csv')
Display the first few rows
print(data.head())
Perform basic analysis
mean = data['column'].mean()
median = data['column'].median()
```
Web scraping allows you to extract data from websites. The BeautifulSoup library simplifies this process:
```python
from bs4 import BeautifulSoup
import requests
Fetch the webpage
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
Extract desired information
titles = soup.find_all('h2', class_='title')
for title in titles:
print(title.text)
```
Automating repetitive tasks can save time and effort. Using the 'os' module, you can perform various operating system tasks:
```python
import os
List files in a directory
files = os.listdir('/path/to/directory')
Rename files
for file in files:
os.rename(file, f'new_{file}')
```
Creating graphical user interfaces (GUIs) is made easy with libraries like Tkinter:
```python
import tkinter as tk
Create a window
window = tk.Tk()
window.title('GUI Example')
Add a label
label = tk.Label(window, text='Hello, World!')
label.pack()
Run the event loop
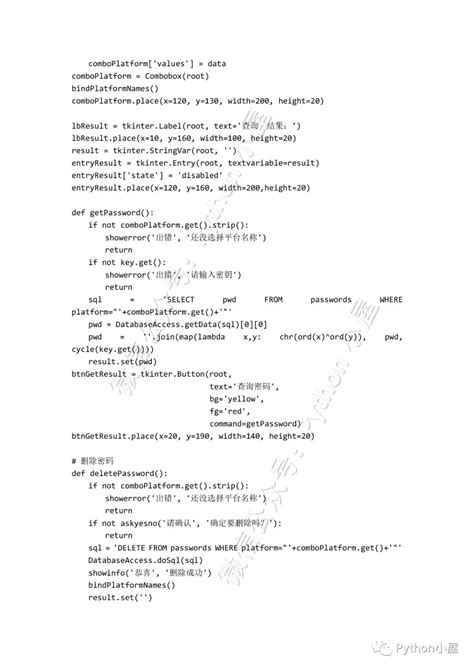
window.mainloop()
```
Proper error handling improves the robustness of your code. Use 'try' and 'except' blocks to handle exceptions:
```python
try:
Risky operation
result = 10 / 0
except ZeroDivisionError:
print('Cannot divide by zero.')
```
These are just a few examples of how Python can be applied in realworld scenarios. Experimenting with different libraries and modules will broaden your understanding and proficiency in Python programming.