Title: Mastering Function Programming in Visual FoxPro (VFP)
Introduction to Function Programming in Visual FoxPro (VFP)
Function Programming in Visual FoxPro (VFP) is a powerful paradigm that allows developers to create modular, reusable code blocks to perform specific tasks. These functions can range from simple calculations to complex data manipulation operations. In this guide, we'll explore the fundamentals of function programming in VFP, discuss best practices, and provide practical examples to illustrate how you can leverage this feature to enhance your development workflow.
Understanding Functions in VFP
In VFP, a function is a named block of code that performs a specific task and returns a value. Functions can accept parameters, which are variables passed to the function to provide input or configure its behavior. They can also have a return value, which is the result of the computation performed by the function.
```foxpro
FUNCTION CalculateArea
LPARAMETERS nWidth, nHeight
RETURN nWidth * nHeight
ENDFUNC
```
In this example, `CalculateArea` is a function that accepts two parameters `nWidth` and `nHeight` and returns their product, which represents the area of a rectangle.
Best Practices for Function Programming in VFP
1.
Modularity
: Break down your code into smaller, reusable functions to promote modularity and maintainability. Each function should perform a single, welldefined task.2.
Parameterization
: Use parameters to make your functions more flexible and versatile. By passing parameters to functions, you can customize their behavior and reuse them in different contexts.3.
Error Handling
: Implement error handling within your functions to gracefully handle unexpected situations and provide meaningful feedback to the caller.4.
Naming Conventions
: Follow consistent naming conventions for your functions to improve readability and maintainability. Use descriptive names that convey the purpose of the function.5.
Documentation
: Document your functions thoroughly, including information about their purpose, parameters, return values, and usage examples. Clear documentation makes it easier for other developers to understand and use your functions.Practical Examples
1.
Calculate Area of a Circle
:```foxpro
FUNCTION CalculateCircleArea
LPARAMETERS nRadius
RETURN PI() * nRadius^2
ENDFUNC
```
2.
Format Phone Number
:```foxpro
FUNCTION FormatPhoneNumber
LPARAMETERS cPhoneNumber
RETURN TRANSFORM(cPhoneNumber, "@R (999) 9999999")
ENDFUNC
```
3.
Fetch Customer Details from Database
:```foxpro
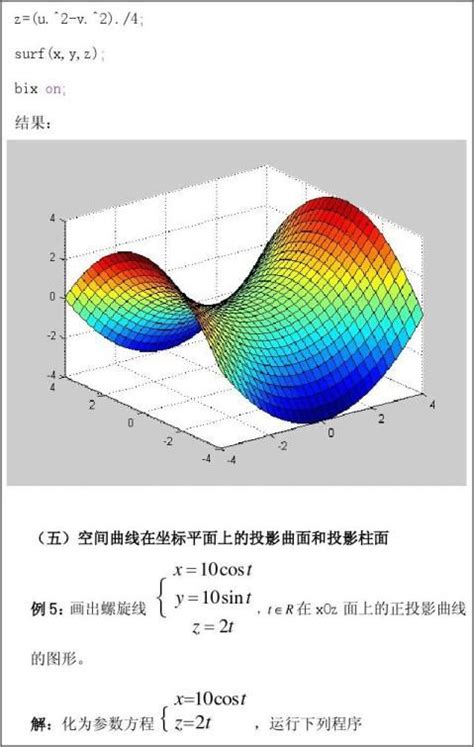
FUNCTION GetCustomerDetails
LPARAMETERS nCustomerId
LOCAL lcQuery
lcQuery = "SELECT * FROM Customers WHERE CustomerID = ?"
PREPARE lcQuery
EXECUTE lcQuery USING nCustomerId
RETURN AGETLAST("Customers")
ENDFUNC
```
Conclusion
Function Programming in Visual FoxPro (VFP) offers developers a powerful tool for creating modular, reusable code blocks to streamline development and improve code maintainability. By following best practices and leveraging practical examples, you can harness the full potential of functions in VFP to build robust and efficient applications. Whether you're performing simple calculations or complex data manipulation operations, mastering function programming in VFP will enhance your development workflow and elevate the quality of your code.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。