Title: Exploring Logs in Programming: A Comprehensive Guide
Logging plays a crucial role in software development, offering insights into the behavior of applications during runtime. Understanding how to effectively utilize logs is essential for debugging, monitoring, and improving the performance of software systems. In this guide, we'll delve into the fundamentals of logging in programming, exploring its significance, best practices, and implementation techniques across various programming languages and environments.
1. What are Logs and Why are They Important?
Logs are records generated by software applications, capturing relevant information about their operation. This information includes errors, warnings, informational messages, and debugging details. Logs serve several key purposes:
Debugging:
Logs help developers identify and troubleshoot issues within the application by providing insights into its execution flow and state.
Monitoring:
Logs enable monitoring the health and performance of applications in realtime, allowing for proactive intervention in case of anomalies or errors.
Auditing and Compliance:
Logs serve as a record of activities performed by the application, aiding in compliance with regulatory requirements and investigation of security incidents. 2. Components of a Log Entry
A typical log entry consists of several components:
Timestamp:
Indicates when the event occurred, aiding in chronological analysis.
Log Level:
Indicates the severity of the message (e.g., DEBUG, INFO, WARN, ERROR, FATAL), facilitating filtering and prioritization.
Message:
Describes the event or condition being logged, providing context for analysis.
Additional Meta
May include contextual information such as the source of the log, thread/process ID, and user/session details. 3. Best Practices for Logging
To maximize the effectiveness of logging, adhere to the following best practices:
Use Appropriate Log Levels:
Choose the appropriate log level for each message to ensure clarity and relevance without overwhelming the logs with unnecessary information.
Avoid Excessive Logging:
Strive for a balance between capturing sufficient information for debugging and monitoring purposes and avoiding excessive verbosity, which can impact performance and readability.
Include Sufficient Context:
Ensure that log messages provide adequate context to facilitate understanding and analysis, including relevant variables, states, and stack traces where applicable.
Secure Sensitive Information:
Avoid logging sensitive data such as passwords, API keys, or personally identifiable information to mitigate security risks.
Centralized Logging:
Consider using centralized logging solutions to aggregate and analyze logs from distributed systems efficiently. 4. Logging in Popular Programming Languages
Python
In Python, logging is facilitated by the builtin `logging` module. Developers can configure loggers, handlers, and formatters to customize logging behavior according to their requirements.
```python
import logging
Configure logging
logging.basicConfig(level=logging.INFO, format='%(asctime)s %(levelname)s %(message)s')
Example usage
logging.info('This is an informational message')
logging.error('This is an error message')
```
Java
Java provides the `java.util.logging` package for logging. Developers can create loggers and handlers to capture and process log messages.
```java
import java.util.logging.Logger;
public class MyClass {
private static final Logger LOGGER = Logger.getLogger(MyClass.class.getName());
public static void main(String[] args) {
LOGGER.info("This is an informational message");
LOGGER.warning("This is a warning message");
}
}
```
JavaScript (Node.js)
In Node.js, developers can use libraries like `winston` or `log4js` for logging. These libraries offer flexibility in configuring loggers, transports, and log levels.
```javascript
const winston = require('winston');
// Configure logger
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
transports: [
new winston.transports.Console(),
new winston.transports.File({ filename: 'app.log' })
]
});
// Example usage
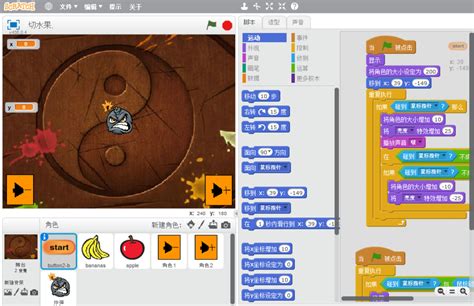
logger.info('This is an informational message');
logger.error('This is an error message');
```
5. Conclusion
Logging is a fundamental aspect of software development, providing invaluable insights into the behavior and performance of applications. By understanding the principles and best practices of logging and leveraging appropriate tools and libraries, developers can effectively debug, monitor, and optimize their software systems. Implementing robust logging mechanisms ensures the reliability, security, and maintainability of software applications across various programming languages and environments.
Whether you're a beginner or an experienced developer, mastering logging techniques is essential for delivering highquality software solutions. Start incorporating logging into your projects today to streamline debugging, enhance monitoring, and elevate the overall quality of your software products.
Happy logging!
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。