Title: Building Password Protected Permissions in C Programming
```html
Building Password Protected Permissions in C Programming
When it comes to programming in C, implementing passwordprotected permissions adds an extra layer of security to your applications. This guide will walk you through the steps to create a basic system for managing permissions with passwords in C programming.
Firstly, you need to define a structure to represent users. Each user should have a username, password, and permissions associated with it. Here's a basic example:
include <stdio.h>
include <string.h>
// Define the structure for a user
struct User {
char username[50];
char password[50];
int permissions; // For simplicity, let's use an integer to represent permissions
};
Next, you'll need functions to authenticate users based on their username and password.
// Function to authenticate a user
int authenticateUser(struct User* users, int numUsers, char* username, char* password) {
for (int i = 0; i < numUsers; i) {
if (strcmp(users[i].username, username) == 0 && strcmp(users[i].password, password) == 0) {
return i; // Return the index of the authenticated user
}
}
return 1; // Return 1 if authentication fails
}
Decide on the permissions you want to grant users and define them as constants.
// Define constants for permissions
define READ_PERMISSION 1
define WRITE_PERMISSION 2
define EXECUTE_PERMISSION 4
Grant permissions to users based on their role or any other criteria you choose.
// Example of granting permissions to a user
users[authenticatedUserIndex].permissions = READ_PERMISSION | WRITE_PERMISSION;
Finally, when users attempt to perform actions, check if they have the necessary permissions.
// Function to check if a user has a specific permission
int hasPermission(struct User* user, int permission) {
return (user>permissions & permission) != 0;
}
Here's a complete example demonstrating the usage of the above functions:
// Define and authenticate users
struct User users[] = {
{"user1", "password1", 0}, // No permissions
{"user2", "password2", READ_PERMISSION}, // Read permission
{"admin", "admin123", READ_PERMISSION | WRITE_PERMISSION | EXECUTE_PERMISSION} // All permissions
};
int numUsers = sizeof(users) / sizeof(users[0]);
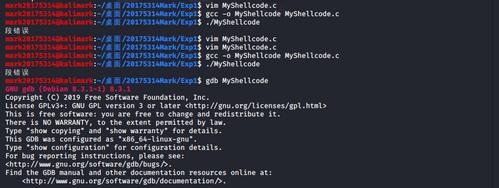
// Authenticate a user
int authenticatedUserIndex = authenticateUser(users, numUsers, "admin", "admin123");
// Check if the authenticated user has write permission
if (authenticatedUserIndex != 1 && hasPermission(&users[authenticatedUserIndex], WRITE_PERMISSION)) {
printf("User has write permission.\n");
} else {
printf("User does not have write permission or authentication failed.\n");
}
With these steps, you can implement a basic passwordprotected permission system in your C programs. Remember to handle password storage securely and consider more advanced security measures for production environments.