Title: The Comprehensive Guide to JavaScript Code
JavaScript (JS) is a versatile programming language widely used for web development. From dynamic website functionality to serverside applications, JS has become integral to modern web development. Below is a comprehensive overview of JavaScript code, covering essential concepts, best practices, and advanced techniques.
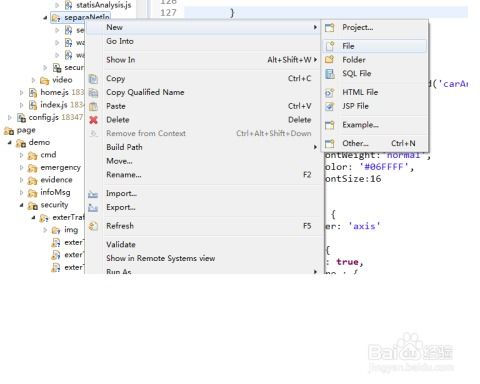
1. Introduction to JavaScript:
JavaScript is a highlevel, interpreted programming language primarily known for its use in web development. It adds interactivity and dynamic behavior to web pages, making them more engaging for users.
2. Basic Syntax and Data Types:
JavaScript syntax is similar to other programming languages like C and Java. Here's an overview of basic syntax and data types:
```javascript
// Variables and Data Types
let num = 10; // Number
let str = "Hello"; // String
let bool = true; // Boolean
let arr = [1, 2, 3]; // Array
let obj = { key: "value" }; // Object
// Conditional Statements
if (num > 5) {
console.log("Number is greater than 5");
} else {
console.log("Number is less than or equal to 5");
}
// Loops
for (let i = 0; i < arr.length; i ) {
console.log(arr[i]);
}
// Functions
function greet(name) {
return "Hello, " name "!";
}
console.log(greet("Alice")); // Output: Hello, Alice!
```
3. DOM Manipulation:
JavaScript is commonly used to manipulate the Document Object Model (DOM) to dynamically update web pages. Here's a basic example:
```html
// DOM Manipulation
const contentDiv = document.getElementById('content');
contentDiv.innerHTML = "
Hello, JavaScript!
";