The nanosleep function in C programming is used to suspend the execution of the calling thread for a specified period of time. It allows for precise control over the timing of a program, which can be crucial in realtime systems, multimedia applications, and other timesensitive scenarios.
Usage
The nanosleep function is typically used in scenarios where a thread needs to wait for a specified duration before proceeding with its operations. It takes the following form:
int nanosleep(const struct timespec *req, struct timespec *rem);
Where:
- req: Points to a
timespec
structure that specifies the requested time for sleep. - rem: If nonNULL, points to a
timespec
structure that receives the remaining sleep time if the call is interrupted by a signal.
Timespec Structure
The timespec
structure is defined as:
struct timespec {
time_t tv_sec; // seconds
long tv_nsec; // nanoseconds
};
This structure allows for the specification of a precise sleep duration in seconds and nanoseconds.
Return Value
The nanosleep
function returns 0 if the requested time has elapsed, or it returns 1 if an error occurs, in which case errno
is set to indicate the nature of the error.
Example
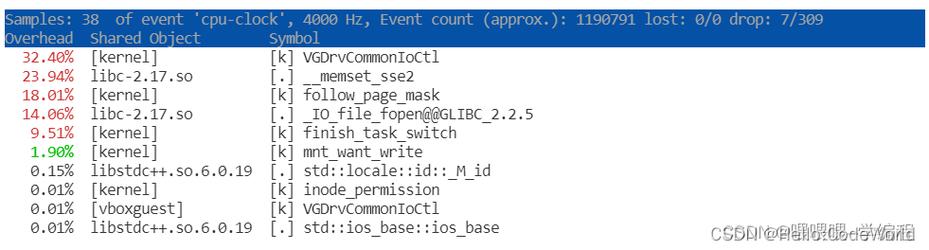
Here is an example of using nanosleep
to pause execution for 1 second:
```c
include
include
int main() {
struct timespec req, rem;
req.tv_sec = 1;
req.tv_nsec = 0;
if (nanosleep(&req, &rem) == 1) {
printf("Sleep was interrupted. Remaining time: %ld.%ld seconds\n", rem.tv_sec, rem.tv_nsec);
} else {
printf("Slept for 1 second\n");
}
return 0;
}
```
In this example, the program attempts to sleep for 1 second. If the sleep is interrupted, it prints the remaining time; otherwise, it indicates that the sleep was successful.
Considerations
When using nanosleep
, it's important to consider the potential for interruptions due to signals. If the sleep is interrupted, the remaining time can be obtained from the rem
parameter, allowing the program to decide how to proceed based on the remaining time.
Additionally, the precision of nanosleep
may vary depending on the underlying operating system and hardware.
Overall, nanosleep
provides a means to precisely control thread execution timing, making it a valuable tool in realtime and timesensitive applications.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。