Title: Understanding the strcpy Function in C Programming
strcpy (string copy) is a standard library function in the C programming language used to copy one string to another. It is defined in the `
Syntax:
```c
char *strcpy(char *destination, const char *source);
```
`destination`: Pointer to the destination array where the content is to be copied.
`source`: String to be copied.
Usage:
```c
include
include
int main() {
char source[] = "Hello, world!";
char destination[20];
// Copying source to destination
strcpy(destination, source);
printf("Source string: %s\n", source);
printf("Destination string: %s\n", destination);
return 0;
}
```
Explanation:
In the example above:
We include the `
Declare two character arrays: `source` and `destination`.
The `strcpy` function is then used to copy the content of `source` to `destination`.
Finally, we print both the source and destination strings.
Considerations:
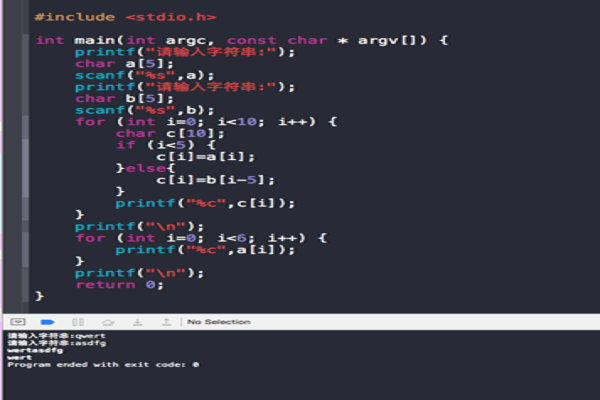
1.
Buffer Overflow
: Ensure that the destination buffer is large enough to hold the source string along with the null terminator. Failure to do so can lead to buffer overflow, resulting in undefined behavior and potential security vulnerabilities.2.
Null Termination
: `strcpy` copies characters from the source string until it encounters a null terminator ('\0'). Therefore, the source string must be nullterminated for proper copying.3.
No Boundary Checks
: `strcpy` does not perform boundary checks. It will continue copying characters from the source string until it encounters the null terminator, potentially causing buffer overflow if the destination buffer is not large enough.4.
Security Risks
: Due to its lack of boundary checks, `strcpy` is susceptible to buffer overflow attacks if not used carefully. Consider using safer alternatives like `strncpy` or functions that perform bounds checking, such as `strcpy_s` (in C11) or `strlcpy` (not standard but available in some libraries).Best Practices:
Always ensure that the destination buffer is large enough to accommodate the source string.
Nullterminate the destination buffer after using `strcpy` to ensure proper string termination.
Consider using safer alternatives if available, especially in scenarios where input size is not guaranteed.
By understanding the `strcpy` function and its considerations, you can effectively utilize it in your C programs while minimizing the risk of errors and vulnerabilities.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。