In Java, a HashMap is a data structure that stores keyvalue pairs. The entrySet
method in HashMap returns a set view of the mappings contained in the map. Each element in this set is a Map.Entry
object, which represents a keyvalue pair.
How to Use entrySet
in HashMap
Here is an example of how you can use the entrySet
method to iterate over the keyvalue pairs in a HashMap:
```java
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
HashMap
hashMap.put("One", 1);
hashMap.put("Two", 2);
hashMap.put("Three", 3);
// Using entrySet to iterate over the keyvalue pairs
for (Map.Entry
System.out.println("Key: " entry.getKey() ", Value: " entry.getValue());
}
}
}
```
When you run this code, it will output:
```
Key: One, Value: 1
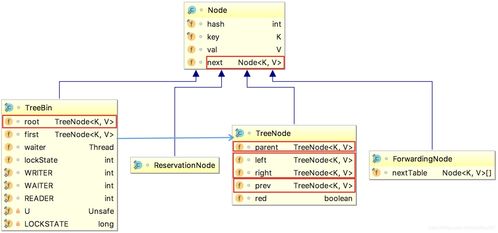
Key: Two, Value: 2
Key: Three, Value: 3
```
Benefits of Using entrySet
Using the entrySet
method provides several benefits when working with HashMap:
entrySet
method provides an efficient way to iterate over keyvalue pairs in a HashMap without the need for separate key and value iterators.
entrySet
, you have access to both keys and values simultaneously, making it convenient to operate on keyvalue pairs.
Map.Entry
object obtained from entrySet
allows you to remove elements safely from the HashMap during iteration. Best Practices for Using entrySet
When using the entrySet
method in a HashMap, consider the following best practices:
Iterator
obtained from the entrySet
to avoid concurrent modification exceptions.
entrySet
compared to using an explicit iterator.
entrySet
.By following these best practices, you can make efficient and safe use of the entrySet
method in HashMap.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。