perror("listen");
include
}
C programming language is commonly used for UNIX network programming due to its efficiency and lowlevel system access. Let's consider a basic example of TCP socket programming in C:
address.sin_addr.s_addr = INADDR_ANY;
exit(EXIT_FAILURE);
Berkeley Sockets API: Widely used for network programming in UNIXlike systems, providing a set of functions for creating and interacting with sockets.
perror("setsockopt");
}
define PORT 8080
Title: Exploring the Essentials of UNIX Network Programming
return 0;
if (bind(server_fd, (struct sockaddr *)&address, sizeof(address)) < 0) {
int opt = 1;
int main() {
}
3. Resource Management: Properly manage system resources such as sockets and memory to prevent resource leaks and system instability.
if ((server_fd = socket(AF_INET, SOCK_STREAM, 0)) == 0) {
send(new_socket, hello, strlen(hello), 0);
perror("accept");
// Creating socket file descriptor
include
This example demonstrates a simple TCP server that listens on port 8080, accepts incoming connections, receives messages from clients, and sends back a "Hello from server" message.
}
UNIX network programming is a domain that involves developing applications that communicate over a network using the UNIX operating system. This discipline encompasses a wide array of concepts, including socket programming, interprocess communication (IPC), and network protocols. Let's delve into the essentials of UNIX network programming and explore its significance in modern computing.
To ensure robust and secure network applications, adhere to the following best practices:
int addrlen = sizeof(address);
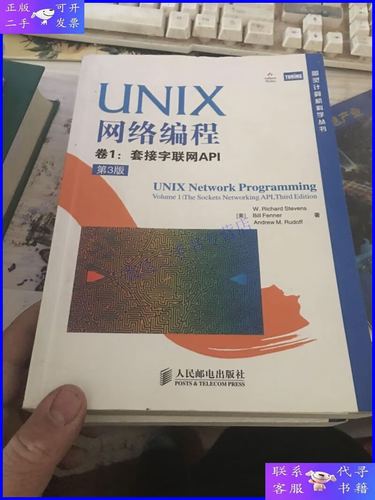
}
1. Error Handling: Always check for errors returned by system calls and handle them gracefully to prevent unexpected crashes.
// Forcefully attaching socket to the port 8080
exit(EXIT_FAILURE);
include
```
UNIX supports various network protocols and APIs for communication. Some of the prominent ones include:
if ((new_socket = accept(server_fd, (struct sockaddr *)&address, (socklen_t*)&addrlen)) < 0) {
4. Security Measures: Implement encryption, authentication, and access control mechanisms to safeguard data and restrict unauthorized access.
char *hello = "Hello from server";
exit(EXIT_FAILURE);
perror("bind failed");
address.sin_family = AF_INET;
address.sin_port = htons(PORT);
}
int server_fd, new_socket;
struct sockaddr_in address;
char buffer[1024] = {0};
exit(EXIT_FAILURE);
if (listen(server_fd, 3) < 0) {
// Forcefully attaching socket to the port 8080
UDP: User Datagram Protocol offers fast, connectionless communication, suitable for applications where realtime data transmission is critical.
Sockets serve as the foundation of UNIX network programming. They provide an interface for communication between processes, either on the same machine or across a network. Sockets are identified by an IP address and a port number, facilitating the establishment of connections between client and server applications.
perror("socket failed");
POSIX IPC: POSIXcompliant InterProcess Communication mechanisms such as message queues, semaphores, and shared memory enable communication between processes on the same system.
exit(EXIT_FAILURE);
In UNIX, sockets are categorized into two main types: stream sockets and datagram sockets. Stream sockets, also known as connectionoriented sockets, offer reliable, bidirectional communication streams, akin to a twoway pipe. Datagram sockets, on the other hand, provide connectionless communication, where data packets are sent individually without any guarantee of delivery or order.
printf("Hello message sent\n");
```c
5. Performance Optimization: Optimize network code for efficiency by minimizing latency, reducing overhead, and utilizing asynchronous I/O techniques where applicable.
include
include
include
printf("Client: %s\n", buffer);
if (setsockopt(server_fd, SOL_SOCKET, SO_REUSEADDR | SO_REUSEPORT, &opt, sizeof(opt))) {
TCP/IP: Transmission Control Protocol/Internet Protocol is the backbone of the Internet and facilitates reliable, connectionoriented communication.
read(new_socket, buffer, 1024);
2. Input Validation: Validate all input received from external sources to mitigate security vulnerabilities such as buffer overflows and injection attacks.
UNIX network programming is a fundamental aspect of modern computing, enabling the development of robust, scalable, and secure network applications. By mastering socket programming, understanding network protocols, and following best practices, developers can create highperformance applications that seamlessly communicate over diverse network environments.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。