Title: Programming DC Motors with C51 Microcontroller
Programming DC motors with the C51 microcontroller, a member of the 8051 family, involves interfacing the microcontroller with the motor and controlling its speed and direction using C programming. This process requires understanding the basics of microcontroller programming, interfacing hardware, and motor control principles. Below is a comprehensive guide to programming DC motors with the C51 microcontroller:
Understanding DC Motors:
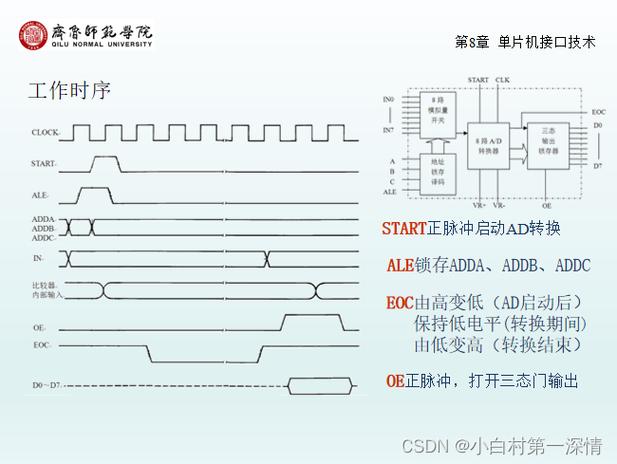
DC motors are widely used in various applications due to their simplicity and controllability. They operate on the principle of electromagnetism, where the interaction between the magnetic field and current produces rotational motion. Key components of a DC motor include the armature (rotating part), commutator, brushes, and a stationary magnetic field.
Interfacing DC Motor with C51 Microcontroller:
To control a DC motor using the C51 microcontroller, you need to interface the microcontroller with motor drivers such as L293D or L298N. These motor drivers act as bridges between the microcontroller and the motor, providing the necessary current and voltage to drive the motor.
The connections between the C51 microcontroller and the motor driver typically involve connecting the output pins of the microcontroller to the input pins of the motor driver, which in turn connect to the DC motor terminals. Additionally, connections for power supply and ground are essential for proper operation.
C51 Programming for Motor Control:
1. Initialize Ports:
Begin by initializing the ports of the C51 microcontroller to define input and output configurations. This step ensures that the microcontroller pins connected to the motor driver are set up correctly for communication.
```c
void initPorts() {
// Configure Port Pins
// Example:
// P1.0 Output for motor direction control
// P1.1 Output for motor PWM signal
// Initialize other ports as needed
}
```
2. Motor Control Functions:
Develop functions to control the direction and speed of the DC motor. This involves sending appropriate signals to the motor driver based on the desired operation.
```c
void setDirection(int direction) {
// Set direction pin based on input (e.g., 0 for clockwise, 1 for counterclockwise)
}
void setSpeed(int speed) {
// Generate PWM signal to control motor speed
}
```
3. Main Program:
In the main program, call the motor control functions based on the application requirements. You can implement various control algorithms such as proportionalintegralderivative (PID) control for precise speed regulation.
```c
int main() {
initPorts(); // Initialize ports
while(1) {
// Control motor direction and speed based on application logic
setDirection(0); // Set direction to clockwise
setSpeed(50); // Set speed to 50% duty cycle (example)
// Implement control algorithms as needed
}
return 0;
}
```
Safety Considerations:
When working with DC motors and microcontrollers, consider the following safety precautions:
Use appropriate power sources and voltage regulators to prevent overloading the microcontroller.
Implement current limiting and protection circuits to safeguard the microcontroller and motor from excessive currents.
Ensure proper insulation and wiring to prevent short circuits and electrical hazards.
Conclusion:
Programming DC motors with the C51 microcontroller involves interfacing the microcontroller with motor drivers and implementing control algorithms in C programming. By understanding the principles of motor operation and microcontroller interfacing, you can develop efficient and reliable motor control systems for various applications.
In summary, mastering the programming techniques outlined above will enable you to create sophisticated control systems for DC motors using the C51 microcontroller. With practice and experimentation, you can further enhance your skills in motor control and embedded systems development.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。