Title: Understanding ASCII in Programming
ASCII, or the American Standard Code for Information Interchange, plays a crucial role in programming, particularly in handling textbased data. Let's delve into what ASCII is, its significance in programming, and how it's utilized across various programming languages.
What is ASCII?
ASCII is a character encoding standard used for representing text in computers and communication equipment. It assigns numeric values to letters, numbers, punctuation marks, and control characters, allowing computers to understand and manipulate textual data.
ASCII Character Set
The ASCII character set comprises 128 characters, each represented by a unique 7bit binary number. These characters include:
Uppercase and lowercase letters
: AZ (6590) and az (97122)
Numbers
: 09 (4857)
Punctuation marks
: ! " $ % & ' ( ) * , . / : ; < = > ? @ [ \ ] ^ _ ` { | } ~
Control characters
: Such as newline (LF), carriage return (CR), tab (TAB), etc.Importance in Programming
1.
Text Processing
: ASCII facilitates reading, writing, and manipulating textbased data in programming languages. Each character is represented by its ASCII code, simplifying text processing tasks.2.
Character Comparison
: In many programming languages, ASCII values are used for comparing characters, sorting strings, and implementing various algorithms.3.
Input/Output Handling
: ASCII is fundamental for handling input/output operations in programs, ensuring compatibility and consistency across different platforms and systems.ASCII in Programming Languages
1. C/C
In C/C , characters are represented using ASCII values. For example:
```c
char ch = 'A';
printf("ASCII value of %c is %d", ch, ch);
```
2. Python
Python also uses ASCII values for character representation. For instance:
```python
char = 'A'
print(f"ASCII value of {char} is {ord(char)}")
```
Best Practices and Considerations
1.
Character Encoding
: While ASCII suffices for basic English text, consider Unicode or other encodings for multilingual applications requiring extended character sets.2.
Platform Independence
: Be mindful of platform differences in ASCII interpretation, especially when dealing with newline characters (\n vs. \r\n).3.
Error Handling
: Handle scenarios where input text contains characters outside the ASCII range gracefully, to avoid unexpected behavior or errors.4.
Security
: Beware of security vulnerabilities such as ASCII injection, especially in web applications where user input is processed.Conclusion
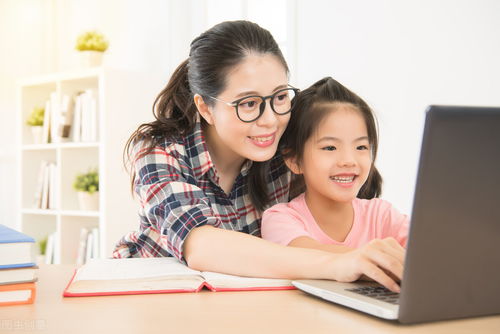
ASCII serves as the foundation for textual data representation and manipulation in programming. Understanding ASCII codes and their usage is essential for effective text processing, input/output handling, and character manipulation across various programming languages and platforms. By adhering to best practices and considering potential pitfalls, developers can leverage ASCII effectively in their applications.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。