socket编程步骤
Title: Understanding Socket Asynchronous Programming in Python
Socket asynchronous programming is a powerful technique used in networking applications to handle multiple connections efficiently. In Python, libraries like `asyncio` and `asyncore` facilitate asynchronous socket programming, enabling developers to build highperformance network applications. Let's delve into the fundamentals and best practices of socket asynchronous programming in Python.
Introduction to Asynchronous Programming
Asynchronous programming allows handling multiple tasks concurrently without blocking the execution flow. In socket programming, this means managing multiple network connections without the need for separate threads or processes per connection.
Understanding Sockets
Sockets are endpoints for communication between two machines over a network. In Python, the `socket` module provides an interface to work with sockets.
Asynchronous Socket Programming in Python
Python offers two primary modules for asynchronous socket programming:
1.
asyncio
: Introduced in Python 3.4, `asyncio` is a library for asynchronous I/O, event loop, and coroutines. It simplifies writing concurrent code and is widely used for network programming.2.
asyncore
: Another asynchronous I/O framework, `asyncore`, is available in Python. It's simpler compared to `asyncio` but provides basic functionality for asynchronous socket programming.Using asyncio for Asynchronous Socket Programming
Here's a basic example of using `asyncio` for asynchronous socket programming:
```python
import asyncio
async def handle_client(reader, writer):
data = await reader.read(100)
message = data.decode()
addr = writer.get_extra_info('peername')
print(f"Received {message} from {addr}")
writer.close()
async def main():
server = await asyncio.start_server(
handle_client, '127.0.0.1', 8888)
async with server:
await server.serve_forever()
asyncio.run(main())
```
In this example:
`asyncio.start_server()` creates a TCP server, and `handle_client` coroutine is called for each client connection.
Inside `handle_client`, it reads data from the client, processes it, and closes the connection.
Using asyncore for Asynchronous Socket Programming
Here's a basic example of using `asyncore` for asynchronous socket programming:
```python
import asyncore
import socket
class EchoHandler(asyncore.dispatcher_with_send):
def handle_read(self):
data = self.recv(8192)
if
self.send(data)
class EchoServer(asyncore.dispatcher):
def __init__(self, host, port):
asyncore.dispatcher.__init__(self)
self.create_socket(socket.AF_INET, socket.SOCK_STREAM)
self.bind((host, port))
self.listen(5)
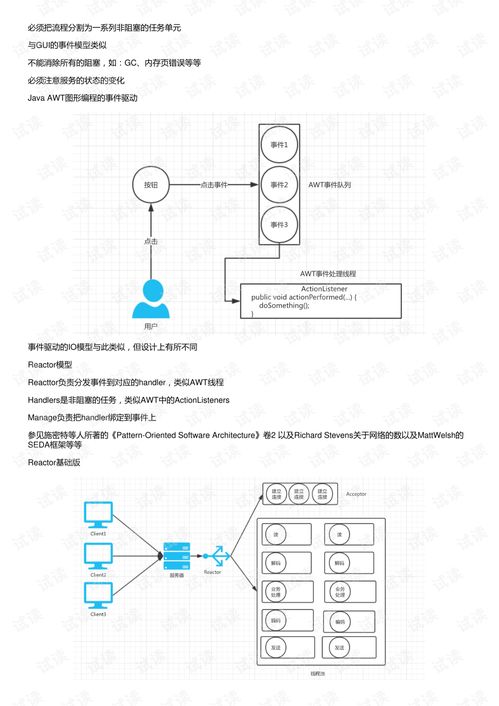
def handle_accept(self):
pair = self.accept()
if pair is not None:
sock, addr = pair
print('Incoming connection from %s' % repr(addr))
handler = EchoHandler(sock)
server = EchoServer('localhost', 8888)
asyncore.loop()
```
In this example:
`EchoServer` listens for incoming connections and creates `EchoHandler` instances to handle each connection.
`EchoHandler` echoes back any data received from the client.
Best Practices for Asynchronous Socket Programming
1.
Use asyncio for Complex Applications
: For complex network applications, `asyncio` provides better support for managing multiple connections and is more featurerich.2.
Keep Operations Nonblocking
: Ensure that all operations within coroutines are nonblocking to avoid blocking the event loop.3.
Handle Errors Gracefully
: Implement error handling to manage exceptions gracefully, ensuring that the application remains stable even in unexpected scenarios.4.
Optimize Performance
: Profile and optimize your code to ensure optimal performance, especially for applications with high traffic volume.5.
Monitor Resource Usage
: Keep an eye on resource usage, such as memory and CPU, to prevent potential bottlenecks and ensure scalability.Conclusion
Socket asynchronous programming in Python, facilitated by libraries like `asyncio` and `asyncore`, empowers developers to create efficient and scalable network applications. By understanding the principles and best practices of asynchronous socket programming, developers can build robust applications capable of handling concurrent connections effectively.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。