Understanding Substr() Function in Programming
The substr() function is a common string manipulation function found in many programming languages. It is used to extract a substring from a larger string based on specified starting and ending positions. Understanding how to use substr() effectively can greatly enhance your ability to manipulate strings in various programming tasks. Let's delve into the details of this function.
The syntax of the substr() function typically involves providing the following parameters:
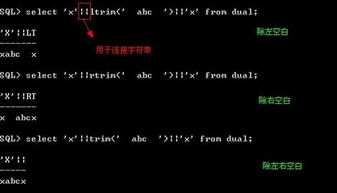
- String: The original string from which the substring will be extracted.
- Start: The position within the string where extraction should begin. This is usually specified as an index.
- Length: The number of characters to extract from the string, starting from the specified start position. This parameter is optional in some programming languages.
Here's a basic representation of the syntax:
substr(string, start, length)
Let's explore some examples of how the substr() function can be used in different programming languages:
JavaScript
// Extracting a substring from index 2 to the endconst str = "Hello, World!";
const result = str.substr(2);
console.log(result); // Output: "llo, World!"
// Extracting a substring of length 5 from index 7
const str2 = "JavaScript";
const result2 = str2.substr(7, 5);
console.log(result2); // Output: "Script"
PHP
// Extracting a substring from index 0 to 5$str = "PHP is a popular scripting language";
$result = substr($str, 0, 5);
echo $result; // Output: "PHP is"
// Extracting a substring starting from index 10 without specifying length
$str2 = "Web development";
$result2 = substr($str2, 10);
echo $result2; // Output: "opment"
When using the substr() function, it's essential to keep the following best practices and tips in mind:
- Understanding Indexing: Remember that string indexing often starts at 0 in many programming languages. Ensure that you're specifying the start position correctly.
- Handling Negative Indices: Some languages support negative indices, which count positions backward from the end of the string. This can be useful for extracting substrings from the end of a string.
- Considering Length: While the length parameter is optional in some languages, specifying it explicitly can make your code more readable and less prone to errors.
- Handling Edge Cases: Be mindful of edge cases, such as when the specified start position is greater than the length of the string or when negative indices are used.
The substr() function is a powerful tool for extracting substrings from larger strings in programming. By understanding its syntax, usage, and best practices, you can manipulate strings more effectively in your code. Whether you're working with JavaScript, PHP, or other programming languages that support this function, mastering substr() will enhance your string manipulation skills and contribute to writing cleaner and more efficient code.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。