max函数编程
Understanding the Max Function in Programming
In programming, the `max` function is a commonly used tool that serves a fundamental purpose: it helps identify the maximum value among a set of values or elements. Whether you're working with numbers, strings, or other data types, the `max` function can streamline your code and simplify tasks involving comparisons. Let's delve deeper into its usage across various programming languages and scenarios.
Basic Syntax and Parameters
The syntax for the `max` function typically varies slightly based on the programming language you're using. However, its core functionality remains consistent: determining the maximum value within a given set.
Python:
In Python, the `max` function has a straightforward syntax:
```python
max_value = max(iterable, *iterables, key=default, default=object)
```
`iterable`: This parameter represents the collection of elements among which you want to find the maximum value.
`*iterables` (optional): You can pass multiple iterables, and the function will return the maximum value among all elements from all iterables.
`key` (optional): A function that defines the comparison key, useful for custom data structures or complex comparisons.
`default` (optional): The value to return if the iterable is empty.
JavaScript:
JavaScript's `Math.max` function behaves similarly:
```javascript
let maxValue = Math.max(...array);
```
`array`: An array containing the elements you want to compare.
Java:
In Java, the `Collections.max` method is commonly used:
```java
T maxValue = Collections.max(collection);
```
`collection`: A collection (e.g., List) containing elements comparable to each other.
Use Cases and Examples
Finding Maximum Numbers:
```python
Python
numbers = [5, 8, 2, 10, 3]
max_number = max(numbers)
print("Maximum number:", max_number) Output: 10
```
```javascript
// JavaScript
let numbers = [5, 8, 2, 10, 3];
let maxNumber = Math.max(...numbers);
console.log("Maximum number:", maxNumber); // Output: 10
```
```java
// Java
List
Integer maxNumber = Collections.max(numbers);
System.out.println("Maximum number: " maxNumber); // Output: 10
```
Comparing Strings:
```python
Python
words = ["apple", "banana", "orange"]
longest_word = max(words, key=len)
print("Longest word:", longest_word) Output: orange
```
```javascript
// JavaScript
let words = ["apple", "banana", "orange"];
let longestWord = words.reduce((a, b) => a.length > b.length ? a : b);
console.log("Longest word:", longestWord); // Output: orange
```
```java
// Java
List
String longestWord = Collections.max(words, Comparator.comparing(String::length));
System.out.println("Longest word: " longestWord); // Output: orange
```
Handling Empty Iterables:
```python
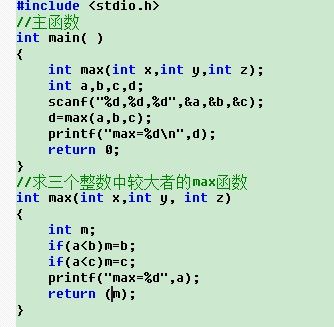
Python
empty_list = []
max_value = max(empty_list, default="List is empty")
print(max_value) Output: List is empty
```
```javascript
// JavaScript
let emptyArray = [];
let maxValue = emptyArray.length > 0 ? Math.max(...emptyArray) : "Array is empty";
console.log(maxValue); // Output: Array is empty
```
```java
// Java
List
Integer maxValue = Collections.max(emptyList, Comparator.naturalOrder());
System.out.println(maxValue); // This will throw NoSuchElementException
```
Conclusion
The `max` function is a versatile tool in programming, enabling you to efficiently find the maximum value within a collection of elements. Whether you're working with numerical data, strings, or custom objects, understanding how to leverage this function can simplify your code and streamline your algorithms. By mastering its usage across different programming languages, you can write more concise and effective code.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。