在编程题中,查找高频词是一个常见的问题,可以通过多种不同的算法和数据结构来解决。下面我们将介绍两种常见的解决方案:哈希表和字典树。
1. 使用哈希表
哈希表是一种使用哈希函数来存储键值对的数据结构。在查找高频词的问题中,可以使用哈希表来统计每个单词出现的次数,然后找到出现频率最高的单词。
以下是使用哈希表解决查找高频词的示例代码(Python):
```python
def find_high_frequency_words(text):
words = text.split()
word_count = {}
for word in words:
word = word.lower() 转换为小写
word_count[word] = word_count.get(word, 0) 1
high_frequency_word = max(word_count, key=word_count.get)
return high_frequency_word
```
通过使用哈希表,我们可以在时间复杂度为 O(n) 的情况下找到高频词,其中 n 为文本中的单词数量。
2. 使用字典树
字典树(Trie)是一种树形数据结构,可以用来存储关联数组,特别适合用于处理字符串。在查找高频词的问题中,可以使用字典树来统计每个单词的出现次数,然后找到出现频率最高的单词。
以下是使用字典树解决查找高频词的示例代码(Python):
```python
class TrieNode:
def __init__(self):
self.children = {}
self.count = 0
def insert_word(root, word):
node = root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.count = 1
def find_high_frequency_word(root, text):
words = text.split()
for word in words:
word = word.lower() 转换为小写
insert_word(root, word)
max_count = 0
high_frequency_word = ''
stack = [root]
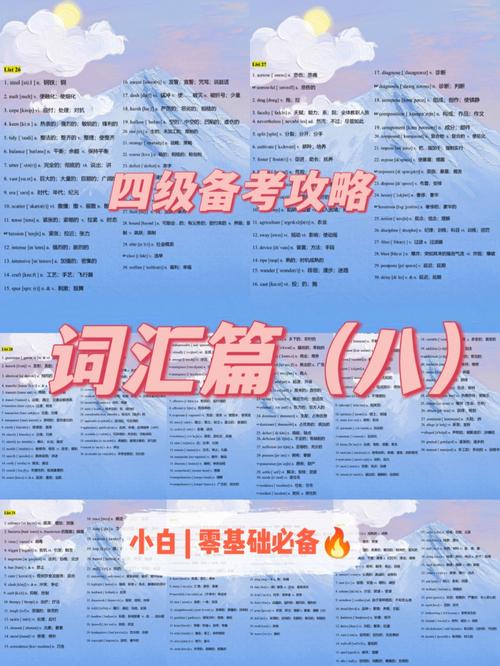
while stack:
node = stack.pop()
for child in node.children.values():
stack.append(child)
if child.count > max_count:
max_count = child.count
high_frequency_word = child
return high_frequency_word
```
通过使用字典树,我们可以在时间复杂度为 O(n) 的情况下找到高频词,其中 n 为文本中的单词数量。
总结
查找高频词是一个常见的编程问题,我们可以使用哈希表或字典树来解决这个问题。哈希表适合于处理大量文本数据,而字典树适合于处理大量单词的情况。
在实际应用中,我们可以根据具体的场景和数据规模选择合适的解决方案,以提高效率和性能。
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。