Title: Introduction to Test Case Scripting in Software Development
In software development, Test Case (TC) scripting plays a crucial role in ensuring the reliability, functionality, and quality of a software product. Test Case scripts are essentially sets of instructions designed to validate whether the software behaves as expected under various conditions. Let's delve into the fundamentals of TC scripting and explore some best practices.
Understanding Test Case Scripting:
Test Case scripting involves the creation of stepbystep procedures to verify the correctness of software functionalities. These scripts are typically written in programming or scripting languages such as Python, Java, or specialized testing frameworks like Selenium for web applications.
Components of a Test Case Script:
1.
Test Case Identifier:
Each TC script should have a unique identifier for easy tracking and reference.2.
Preconditions:
Conditions that must be met before executing the test case.3.
Test Steps:
Detailed instructions to be followed during the test execution.4.
Expected Results:
The outcomes or behaviors expected from the software after executing the test steps.5.
Actual Results:
The actual outcomes observed during test execution, recorded for comparison with expected results.6.
Status:
Indicates whether the test case passed, failed, or needs further investigation.7.
Remarks:
Additional comments or notes related to the test case execution.Best Practices for TC Scripting:
1.
Clarity and Simplicity:
Write clear and concise test steps that are easy to understand and execute.2.
Modularity:
Break down complex test cases into smaller, manageable modules for better maintainability and reusability.3.
DataDriven Testing:
Parameterize test inputs to cover a wider range of scenarios without duplicating test cases.4.
Error Handling:
Anticipate and handle potential errors gracefully within the test scripts to ensure robustness.5.
Documentation:
Maintain thorough documentation for each test case, including purpose, inputs, outputs, and dependencies.6.
Version Control:
Store test scripts in a version control system like Git to track changes and collaborate effectively.7.
CrossBrowser/Platform Testing:
Consider the diversity of user environments and ensure compatibility across different browsers and platforms.8.
Continuous Integration:
Integrate TC scripts with CI/CD pipelines to automate testing and ensure early detection of issues.Example Test Case Script (Using Python and Selenium):
```python
import unittest
from selenium import webdriver
class GoogleSearchTest(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Chrome()
def test_google_search(self):
driver = self.driver
driver.get("https://www.google.com")
search_box = driver.find_element_by_name("q")
search_box.send_keys("Test Automation")
search_box.submit()
search_results = driver.find_elements_by_css_selector("h3")
self.assertTrue(len(search_results) > 0, "Search results not found")
def tearDown(self):
self.driver.quit()
if __name__ == "__main__":
unittest.main()
```
Conclusion:
Test Case scripting is an indispensable aspect of software testing and quality assurance. By following best practices and leveraging appropriate tools and frameworks, teams can effectively design, automate, and execute test cases to ensure the reliability and functionality of their software products. Embracing a systematic approach to TC scripting contributes to the overall success of software development projects.
References:
[Selenium Documentation](https://www.selenium.dev/documentation/en/)
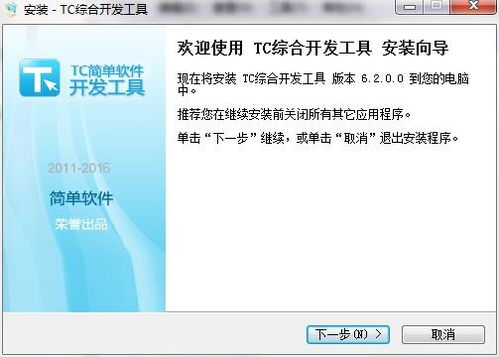
[Python unittest Documentation](https://docs.python.org/3/library/unittest.html)
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。