探索有趣的编程代码
编程世界中充满了许多有趣的代码和技巧,下面我将分享一些有趣的编程代码示例,让你一窥编程的乐趣。
1. 生成斐波那契数列
```python
def fibonacci(n):
a, b = 0, 1
for _ in range(n):
yield a
a, b = b, a b
输出前10个斐波那契数
print(list(fibonacci(10)))
```
这段代码展示了如何使用 Python 的生成器函数生成斐波那契数列,通过 yield 关键字实现惰性计算。
2. ASCII艺术
```python
print(r"""
__ __ _ _ _
| \/ (_) | | (_)
| \ / |_ _____ _____ _ __ | |_ _ ___ _ __
| |\/| | |/ _ \ \ /\ / / _ \| '_ \ | __| |/ _ \| '_ \
| | | | | __/\ V V / (_) | | | || |_| | (_) | | | |
|_| |_|_|\___| \_/\_/ \___/|_| |_(_)__|_|\___/|_| |_|
""")
```
这段代码通过打印特定的字符串实现了简单的 ASCII 艺术输出,为控制台程序增添了趣味性。
3. 用递归方式计算阶乘
```javascript
function factorial(n) {
if (n === 0 || n === 1) {
return 1;
} else {
return n * factorial(n 1);
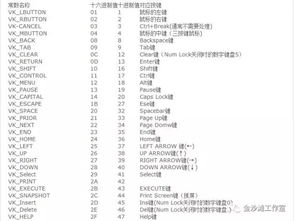
}
}
// 输出10的阶乘
console.log(factorial(10));
```
这是一个使用递归方式计算阶乘的 JavaScript 函数,展示了递归在数学计算中的妙用。
4. 螺旋矩阵生成器
```python
def generate_spiral_matrix(n):
matrix = [[0] * n for _ in range(n)]
dirs = [(0, 1), (1, 0), (0, 1), (1, 0)]
cur_dir = 0
cur_pos = (0, 0)
for i in range(1, n * n 1):
matrix[cur_pos[0]][cur_pos[1]] = i
next_pos = (cur_pos[0] dirs[cur_dir][0], cur_pos[1] dirs[cur_dir][1])
if 0 <= next_pos[0] < n and 0 <= next_pos[1] < n and matrix[next_pos[0]][next_pos[1]] == 0:
cur_pos = next_pos
else:
cur_dir = (cur_dir 1) % 4
cur_pos = (cur_pos[0] dirs[cur_dir][0], cur_pos[1] dirs[cur_dir][1])
for row in matrix:
print(row)
生成 5x5 的螺旋矩阵
generate_spiral_matrix(5)
```
这段 Python 代码通过模拟螺旋矩阵填充的方式生成了一个螺旋矩阵,展示了编程在数学和算法领域的应用。
5. 用CSS实现动态旋转的立方体
```html
.container {
perspective: 800px;
}
.cube {
position: relative;
width: 200px;
transformstyle: preserve3d;
animation: rotateCube 5s infinite linear;
}
.face {
position: absolute;
width: 200px;
height: 200px;
lineheight: 200px;
textalign: center;
fontsize: 24px;
color: white;
}
.face1 { backgroundcolor: 3498db; transform: rotateY(0deg) translateZ(100px); }
.face2 { backgroundcolor: e74c3c; transform: rotateY(90deg) translateZ(100px); }
.face3 { backgroundcolor: 2ecc71; transform: rotateY(180deg) translateZ(100px); }
.face4 { backgroundcolor: f1c40f; transform: rotateY(90deg) translateZ(100px); }
.face5 { backgroundcolor: 9b59b6; transform: rotateX(90deg) translateZ(100px); }
.face6 { backgroundcolor: 34495e; transform: rotateX(90deg) translateZ(100px); }
@keyframes rotateCube {
from { transform: rotateY(0); }
to { transform: rotateY(360deg); }
}