Title: Coding Exercises for Cab Management System
1.
Problem Statement 1: Design Classes for Cab Management System
In a cab management system, there are different entities like Cab, Driver, Customer, and Ride. Design the classes for these entities along with their attributes and methods.
Solution:
```python
class Cab:
def __init__(self, cab_id, model, available):
self.cab_id = cab_id
self.model = model
self.available = available True if available, False if booked
class Driver:
def __init__(self, driver_id, name, rating):
self.driver_id = driver_id
self.name = name
self.rating = rating
class Customer:
def __init__(self, customer_id, name, mobile):
self.customer_id = customer_id
self.name = name
self.mobile = mobile
class Ride:
def __init__(self, ride_id, cab, customer, distance, fare):
self.ride_id = ride_id
self.cab = cab
self.customer = customer
self.distance = distance
self.fare = fare
```
2.
Problem Statement 2: Implement Cab Booking and Ride Calculation
Write a function to book a cab for a customer based on the distance to be traveled. The fare should be calculated based on the distance traveled and any other relevant factors. Also, update the availability of the cab after booking.
Solution:
```python
def book_cab(customer, distance):
available_cabs = [cab for cab in cabs if cab.available]
if available_cabs:
cab = available_cabs[0] Choose the first available cab for booking
fare = distance * 10 Assuming fare calculations based on distance
ride_id = generate_ride_id() Assume a function to generate a unique ride ID
ride = Ride(ride_id, cab, customer, distance, fare)
cab.available = False Mark the cab as booked
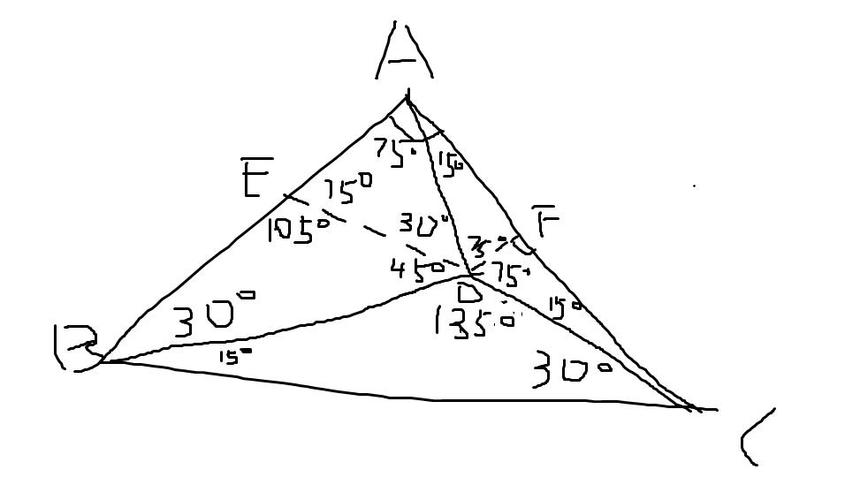
return ride
else:
return "No cabs available"
```
3.
Problem Statement 3: Rating System for Drivers
Design a rating system for drivers where customers can provide a rating (out of 5) after completing a ride. Update the driver's rating based on the average of all the ratings received.
Solution:
```python
def update_driver_rating(driver, new_rating):
total_rating = driver.rating
total_rides = 1 Assuming the driver has completed at least one ride
new_average_rating = (total_rating new_rating) / (total_rides 1)
driver.rating = new_average_rating
```
4.
Problem Statement 4: Adding Realtime Tracking Feature
Implement a realtime tracking feature for customers to track the location of the booked cab until it reaches them.
Solution:
This feature involves integration with mapping and location services such as Google Maps API or Mapbox. Depending on the chosen service, the implementation would involve setting up realtime location updates for the cab and providing a tracking interface for the customers.
These coding exercises simulate realworld scenarios in a cab management system and focus on class design, booking logic, rating systems, and realtime tracking. They provide a practical opportunity to implement key features of a cab management system.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。